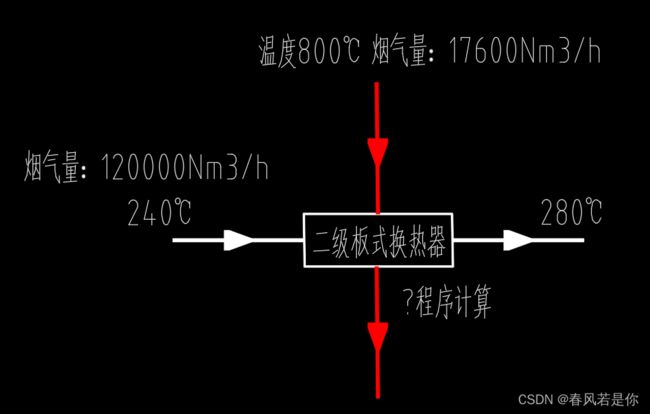
"""
Created on Thu Nov 30 11:23:12 2023
计算两股不同流量的气体,通过换热器换热后,高温气体的出口温度
(煤烟二级,计算煤烟二级热侧出口温度)
------------------------------------------------
已知:冷测烟气量、出入口温度,热侧烟气量、热侧入口温度
求热侧出口温度
保证热出比冷进高15℃
------------------------------------------------
@author: xujifa
"""
import sympy
class Com_two_hot_out_temperature():
def __init__(self, cold_in_fl, cold_in_tem,cold_out_tem,hot_in_fl,hot_in_tem,name):
self.cold_in_fl = cold_in_fl
self.cold_in_tem = cold_in_tem
self.cold_out_fl = cold_in_fl
self.cold_out_tem = cold_out_tem
self.hot_in_fl = hot_in_fl
self.hot_in_tem = hot_in_tem
self.hot_out_fl = cold_in_fl
self.name = str(name)
self.cha = 15
print(f"以下计算为 {self.name} 的参数")
def ex_eff(self):
if self.hot_in_tem > 400:
return 0.94
else:
return 0.965
def com_heat(self,fl,tem):
fl = fl/0.0224
tem = tem + 273.15
cp = 7.22e-10*pow(tem,3) - 5.937e-06*pow(tem,2) + 0.01707 *tem + 26.52
heat = cp*fl*tem
return heat
def ex_heat(self):
cold_in_heat = self.com_heat(self.cold_in_fl,self.cold_in_tem)
cold_out_heat = self.com_heat(self.cold_out_fl,self.cold_out_tem)
heat = cold_out_heat - cold_in_heat
print(f"***{self.name} @ 冷测热量:{heat} J/h",)
print(f"***{self.name} @ 冷测热量:{heat/3600/1000} KW",)
return heat
def com_hot_out_heat(self):
hot_in_heat = self.com_heat(self.hot_in_fl,self.hot_in_tem)
print(f"+++{self.name} @ 热侧入口携带热量:{hot_in_heat} J/h")
cold_ex_heat = self.ex_heat()/self.ex_eff()
hot_out_heat = (hot_in_heat - cold_ex_heat)/(self.hot_in_fl/0.0224)
print(f"+++{self.name} @ 热侧出口所需热量:{hot_out_heat} J/h")
return hot_out_heat
def sol_tem(self,h):
tem = sympy.Symbol("tem")
s = sympy.solve(7.22e-10*pow(tem,4) - 5.937e-06*pow(tem,3) + 0.01707 *tem*tem + 26.52*tem-h)
print(f'↓↓↓***{self.name} @ 计算结果温度 K-待筛选***↓↓↓')
print(s)
print(f'↑↑↑***{self.name} @ 计算结果温度 K-待筛选***↑↑↑')
return s
def post_sol(self,data):
for num in data:
if "I" not in str(num):
if num > 0:
print(f"{self.name}@热侧出口温度 {round(num-273.15,2)} ℃")
print(f"以上计算为 {self.name} 的参数")
return round(num-273.15,2)
else:
print("solve-复数")
return -911111111
def getdata(self):
hot_out_tem = self.post_sol(self.sol_tem(self.com_hot_out_heat()))
print(f"热侧出口温度为{hot_out_tem}-经过方程求解")
print("---------------------------------")
print(f"{self.name}边界参数")
print(f"{self.name}-冷测:\n1、流量{self.cold_in_fl}Nm3/h\n2、入口温度{self.cold_in_tem}℃\n3、出口温度{self.cold_out_tem}℃\n4、温差{self.cold_out_tem-self.cold_in_tem}℃")
print(f"{self.name}-热侧:\n1、流量{self.hot_in_fl}Nm3/h\n2、入口温度{self.hot_in_tem}℃\n3、出口温度{hot_out_tem}℃\n4、温差{self.hot_in_tem-hot_out_tem}℃")
print("---------------------------------")
return self.cold_out_fl,self.cold_out_tem,self.hot_out_fl,hot_out_tem
if __name__ == "__main__":
Com_two_hot_out_temperature(122000, 240, 280, 15000,200,"二级换热器").getdata()