1.是什么?
是运行在java服务器端的小程序,,用于接收和响应客户端的请求和响应,要想实现Servlet功能,可以实现Servlet接口或者继承HTTPserv
let和GenericServlet,每次请求都会执行service()核心方法
Servlet的三种实现
(1)实现Servlet,实现所有抽象方法,最大程度支持了自定义
(2)继承GenericService抽象类,必须重写Service方法,其他方法可以选择重写
(3)继承HTTPService,重写doget和dopost方法
注意:继承GenericServlet要重写service方法
2.关系视图
HTTPServlet------继承—>>GenericService------实现—>>Servlet接口
3.执行流程
客户端浏览器发起请求---------Tomcat服务器------>>解析请求地址(URL),找到对应的应用--------->>找到应用的web.xml-------->>请求解析资源地址(URI)-------->>找到对应的资源--------->>响应给客户端浏览器
图解:
4.Servlet的生命周期
出生----->>或者------>>死亡 对象的创建到销毁
出生:第一次请求到达Servlet,对象创建出来,并初始化成功,只出生一次,放在内存中
或者:服务器提供服务的整个过程,每次都执行Service方法
死亡:服务器宕机,停止,就会死亡
代码:
public class Servlet__Demo01 extends HttpServlet {
@Override
public void init() throws ServletException {
System.out.println("我创建了....");
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("执行了.....");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
@Override
public void destroy() {
System.out.println("我挂了.....");
}
}
web.xml:
Servlet__Demo01 com.itheima.servlet.Servlet__Demo01 1 Servlet__Demo01 /Demo01 load-on-startup标签可以修改Servlet的创建时机,整数代表服务器加载时创建,值越小,优先级越高,负数或不写代表第一次访问创建 5.Servlet的线程安全问题 Serlet是但实例,在应用中只有一个对象,所以涉及线程安全问题,所以不能定义成员变量,同步代码块会影响servlet 6.Servlet映射方式 (1).指名道姓方式,访问资源必须和映射完全相同 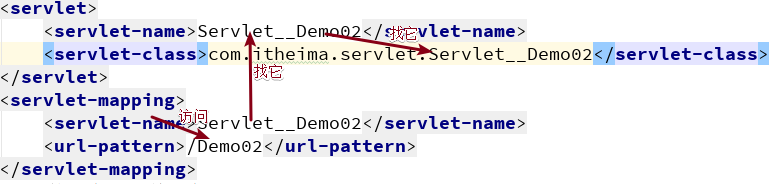 地址栏: 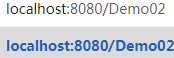 (2).通配符方式 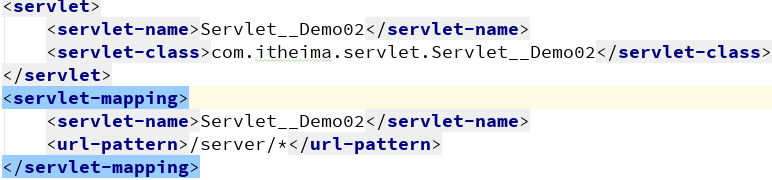 地址栏:  (3).通配符加固定格式结尾 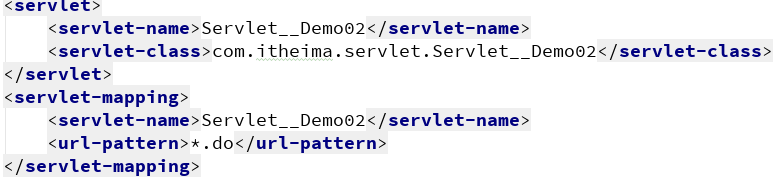 地址栏:  三种方式的优先级顺序--------->>越是精准优先级越高7.ServletConfig介绍
是Servlet配置参数对象,提供初始化配置
作用:在Servlet初始化时,把一些配置信息传递给Servlet
生命周期:和Servlet相同
初始化配置是怎么传递的
它是通过Tomcat把初始化配置封装成对象,因为init()等....都是Tomcat在调用
配置方式
在,通过标签类配置,有两个子标签
:初始化key
:初始化value
可以配置多个初始化配置,但是要多写
代码:
Servlet__Demo03 com.itheima.servlet.Servlet__Demo03 encoding UTF-8 desc This is Desc Servlet__Demo03 *.do 常用方法: 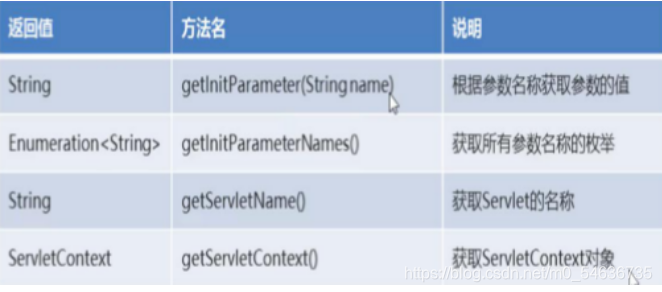
代码:
public class Servlet__Demo03 extends HttpServlet {
private ServletConfig config;
//要把ServletConfig对象传给init()方法
@Override
public void init(ServletConfig config) throws ServletException {
//获取初始化参数,根据key获取value
String encodingvalue = config.getInitParameter("encoding");
System.out.println(encodingvalue);
System.out.println("-------------");
//获取初始化所有key的集合
Enumeration<String> keys = config.getInitParameterNames();
//遍历
while (keys.hasMoreElements()) {
//拿出所有的key
String key = keys.nextElement();
// 根据key获取value
String value = config.getInitParameter(key);
System.out.println(key + "," + value);
}
System.out.println("-----------------");
// 获取servlet名称
String servletName = config.getServletName();
System.out.println("-------------");
//获取servletContext对象
ServletContext servletContext = config.getServletContext();
System.out.println(servletContext);
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("我执行了............");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
}
8.ServletContext介绍
它是上下文对象,每个应用只有一个
作用:可以配置全局初始化参数
可以实现Servlet之间的数据共享
配置:
在标签中,通过来配置,有两个子标签
:全局初始化key
:全局初始化value
可以配置多个,但是要写多个
代码:
Servlet__Demo04 com.itheima.servlet.Servlet__Demo04 Servlet__Demo04 /servlet/* globalEncoding UTF-8 ServletContextInfo This is application 常用方法: 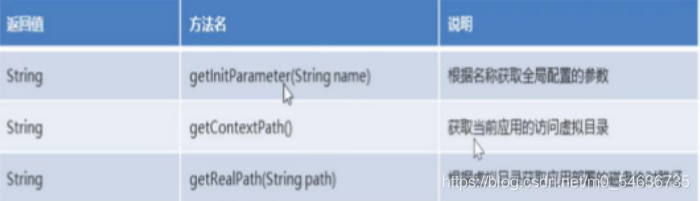 代码:
public class Servlet__Demo04 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// 获取ServletContext对象
ServletContext servletContext = getServletContext();
//根据key,获取value
String globalEncoding = servletContext.getInitParameter("globalEncoding");
System.out.println(globalEncoding);
//获取当前访问虚拟目录
String contextPath = servletContext.getContextPath();
System.out.println(contextPath);
// 获取应用部署磁盘的绝对路径------>>这是拿到部署Tomcat的绝对路径
//java中不能硬编码,但是需要绝对路径,就采取此种方式
String realPath = servletContext.getRealPath("/");
System.out.println(realPath);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
}
注意:
servletContext.getRealPath("/");拿到的是部署Tomcat的绝对路径
9.域对象
作用范围,就是做数据共享的,不同的域对象,共享的范围也不一样,在Servlet规范中,共有四个域对象
其中ServletContext是其中一个,也叫application域,是最大的域,可以实现整个应用之间的数据共享
四大域对象通用的三个方法
(1).setAttribute
设置共享域数据
(2)getAttribute
获取共享域数据
(3)removeAttribute
删除共享域数据
代码:
设置 删除共享数据
```java
public class ServletDemo03 extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ServletContext context = getServletContext();
//设置共享数据
// context.setAttribute("username","zhangsan");
//删除共享数据
context.removeAttribute("username");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
}
获取共享数据
```java
public class ServletDemo03bar extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
ServletContext context = getServletContext();
// 获取ServletContext共享域数据
Object username = context.getAttribute("username");
System.out.println(username);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
}
10.注解开发Servlet
3.0以后可以使用注解
自动注解开发
再类上使用@WebServlet("/访问资源的路径")
11.案例
在web,xml中配置
<!-- 修改默认主页-->
<welcome-file-list>
<welcome-file>/addStudent.html</welcome-file>
</welcome-file-list>
浏览器访问web,xml会先访问/addStudent.html
在html中制作表单
```java
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>添加学生</title>
</head>
<body>
<form action="/stu/StudentServlet" method="get" autocomplete="off">
<input type="text" name="username"><br/>
<input type="text" name="age"><br/>
<input type="text" name="score"><br/>
<button type="submit">保存</button>
</form>
</body>
</html>
当表单提交数据后 action会指向Servlet配置 /stu为虚拟路径 /StudentServlet为映射的资源路径
```java
<!-- 配置servlet-->
<servlet>
<servlet-name>studentServlet</servlet-name>
<servlet-class>com.itheima.servlet.StudentServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>studentServlet</servlet-name>
<url-pattern>/StudentServlet</url-pattern>
</servlet-mapping>
通过映射找到资源
```java
public class StudentServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取表单中的数据
String username = req.getParameter("username");
String age = req.getParameter("age");
String score = req.getParameter("score");
//通过输出流将数据保存到stu.txt文件中
BufferedWriter bw = new BufferedWriter(new FileWriter("d:\\stu.txt", true));
bw.write(username+","+age+","+score);
bw.newLine();
bw.close();
//给出浏览器响应,打印响应
PrintWriter pw = resp.getWriter();
pw.write("sccess...");
pw.close();
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req,resp);
}
}