springboot整合elasticsearch
引用依赖
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0modelVersion>
<groupId>com.ohb.springbootgroupId>
<artifactId>springboot-esartifactId>
<version>1.0-SNAPSHOTversion>
<properties>
<maven.compiler.source>8maven.compiler.source>
<maven.compiler.target>8maven.compiler.target>
<project.knife4j.version>4.0.0project.knife4j.version>
properties>
<parent>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-parentartifactId>
<version>2.6.13version>
parent>
<dependencies>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-data-elasticsearchartifactId>
dependency>
<dependency>
<groupId>org.projectlombokgroupId>
<artifactId>lombokartifactId>
<version>1.18.24version>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-testartifactId>
<scope>testscope>
dependency>
<dependency>
<groupId>junitgroupId>
<artifactId>junitartifactId>
<version>4.12version>
<scope>testscope>
dependency>
dependencies>
project>
配置文件(application.yml)
spring:
elasticsearch:
uris: http://192.168.198.129:9200
data:
elasticsearch:
repositories:
enabled: true
application:
name: springboot-es
server:
port: 9070
knife4j:
enable: true
openapi:
title: knife4测试elasticsearch
description: 测试接口
email: [email protected]
concat: ohb
配置类
package com.ohb.springboot.es.config;
import org.elasticsearch.client.RestHighLevelClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.data.elasticsearch.client.ClientConfiguration;
import org.springframework.data.elasticsearch.client.RestClients;
import org.springframework.data.elasticsearch.config.AbstractElasticsearchConfiguration;
public class ElasticsearchConfig extends AbstractElasticsearchConfiguration {
@Value("${spring.elasticsearch.uris}")
private String uris;
@Override
public RestHighLevelClient elasticsearchClient() {
ClientConfiguration configuration=
ClientConfiguration.builder()
.connectedTo(uris)
.build();
return RestClients.create(configuration).rest();
}
}
文档类
package com.ohb.springboot.es.entity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.DateFormat;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
import java.math.BigDecimal;
import java.time.LocalDate;
@Data
@NoArgsConstructor
@AllArgsConstructor
@Document(indexName = "book_index",createIndex = true)
public class BookDoc {
@Id
@Field(type = FieldType.Text)
private String id;
@Field(type = FieldType.Text)
private String title;
@Field(type = FieldType.Text)
private String author;
@Field(type = FieldType.Text)
private String publisher;
@Field(type = FieldType.Double)
private BigDecimal price;
@Field(type = FieldType.Text)
private String content;
@Field(type = FieldType.Date,format = DateFormat.year_month_day)
@JsonFormat(pattern = "yyyy-MM-dd",timezone = "GMT+8")
private LocalDate publishDate;
}
DTO类
package com.ohb.springboot.es.dto;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.Date;
@Data
@NoArgsConstructor
@AllArgsConstructor
@ApiModel("DTO")
public class HttpResp {
@ApiModelProperty("DTO返回代码")
private int code;
@ApiModelProperty("DTO返回信息")
private String msg;
@ApiModelProperty("dto返回时间")
private Date time;
@ApiModelProperty("dto返回数据")
private Object results;
}
定义elasticsearch操作数据接口
package com.ohb.springboot.es.dao;
import com.ohb.springboot.es.entity.BookDoc;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
public interface IBookRepository extends ElasticsearchRepository<BookDoc,String> {
}
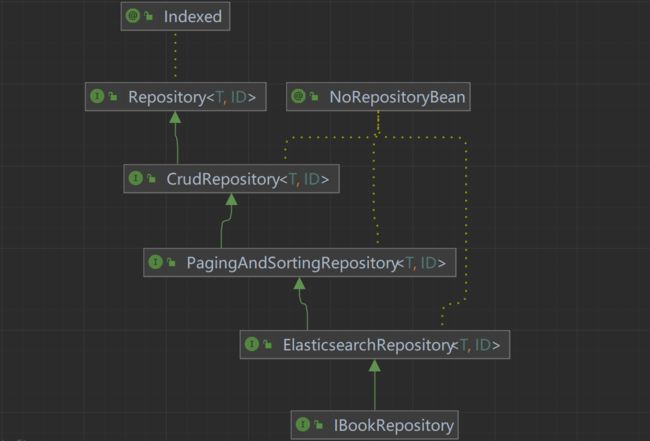
服务层接口(service)
接口
package com.ohb.springboot.es.service;
import com.ohb.springboot.es.entity.BookDoc;
import java.util.List;
public interface IBookService {
List<BookDoc> findAll();
void addBookDoc(BookDoc bookDoc);
}
实现类
package com.ohb.springboot.es.service.impl;
import com.ohb.springboot.es.dao.IBookDocRepository;
import com.ohb.springboot.es.entity.BookDoc;
import com.ohb.springboot.es.service.IBookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class BookServiceImpl implements IBookService {
@Autowired
private IBookDocRepository ibdr;
@Override
public List<BookDoc> findAll() {
List<BookDoc> list = new ArrayList<>();
ibdr.findAll().forEach((bc) -> list.add(bc));
return list;
}
@Override
public void addBookDoc(BookDoc bookDoc) {
}
}
控制层(controller)
package com.ohb.springboot.es.controller;
import com.ohb.springboot.es.dto.HttpResp;
import com.ohb.springboot.es.service.IBookService;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Date;
@Api(tags = "图书接口类")
@RestController
@RequestMapping("/book/api")
public class BookController {
@Autowired
private IBookService ibs;
@ApiOperation("查询所有图书信息")
@GetMapping("/findAllBooks")
public HttpResp findAllBooks() {
return new HttpResp(20001, "查询所有图书成功", new Date(), ibs.findAll());
}
}
测试
package com.ohb.springboot.es.dao;
import com.ohb.springboot.es.entity.Book;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import java.math.BigDecimal;
import java.time.LocalDate;
import static org.junit.Assert.*;
@SpringBootTest
@RunWith(SpringJUnit4ClassRunner.class)
public class IBookRepositoryTest {
@Autowired
private IBookRepository bookRepository;
@Test
public void saveBook(){
bookRepository.save(new BookDoc("wnhz001","西游记","吴承恩","神话出版社", BigDecimal.valueOf(33.5),"历经千险,终成大道", LocalDate.now()));
}
@Test
public void findAll(){
bookRepository.findAll().forEach(System.out::println);
}
}
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.6.13)
2023-03-08 17:25:50.048 INFO 6936 --- [ main] c.o.s.es.dao.IBookRepositoryTest : Starting IBookRepositoryTest using Java 1.8.0_311 on XTZJ-20220114OE with PID 6936 (started by Administrator in D:\wnhz-workspace\springboot\springboot-es)
2023-03-08 17:25:50.053 INFO 6936 --- [ main] c.o.s.es.dao.IBookRepositoryTest : No active profile set, falling back to 1 default profile: "default"
2023-03-08 17:25:51.683 INFO 6936 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Bootstrapping Spring Data Elasticsearch repositories in DEFAULT mode.
2023-03-08 17:25:51.824 INFO 6936 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Finished Spring Data repository scanning in 128 ms. Found 1 Elasticsearch repository interfaces.
2023-03-08 17:25:51.840 INFO 6936 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Bootstrapping Spring Data Reactive Elasticsearch repositories in DEFAULT mode.
2023-03-08 17:25:51.847 INFO 6936 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Finished Spring Data repository scanning in 6 ms. Found 0 Reactive Elasticsearch repository interfaces.
2023-03-08 17:25:58.622 WARN 6936 --- [/O dispatcher 1] org.elasticsearch.client.RestClient : request [GET http://192.168.198.129:9200/] returned 1 warnings: [299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "Elasticsearch built-in security features are not enabled. Without authentication, your cluster could be accessible to anyone. See https://www.elastic.co/guide/en/elasticsearch/reference/7.17/security-minimal-setup.html to enable security."]
2023-03-08 17:25:58.910 WARN 6936 --- [ main] org.elasticsearch.client.RestClient : request [HEAD http://192.168.198.129:9200/book_index?ignore_throttled=false&ignore_unavailable=false&expand_wildcards=open%2Cclosed&allow_no_indices=false] returned 2 warnings: [299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "Elasticsearch built-in security features are not enabled. Without authentication, your cluster could be accessible to anyone. See https://www.elastic.co/guide/en/elasticsearch/reference/7.17/security-minimal-setup.html to enable security."],[299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "[ignore_throttled] parameter is deprecated because frozen indices have been deprecated. Consider cold or frozen tiers in place of frozen indices."]
2023-03-08 17:25:59.096 INFO 6936 --- [ main] c.o.s.es.dao.IBookRepositoryTest : Started IBookRepositoryTest in 10.118 seconds (JVM running for 13.203)
2023-03-08 17:25:59.977 WARN 6936 --- [ main] org.elasticsearch.client.RestClient : request [POST http://192.168.198.129:9200/book_index/_search?typed_keys=true&max_concurrent_shard_requests=5&ignore_unavailable=false&expand_wildcards=open&allow_no_indices=true&ignore_throttled=true&search_type=query_then_fetch&batched_reduce_size=512] returned 2 warnings: [299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "Elasticsearch built-in security features are not enabled. Without authentication, your cluster could be accessible to anyone. See https://www.elastic.co/guide/en/elasticsearch/reference/7.17/security-minimal-setup.html to enable security."],[299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "[ignore_throttled] parameter is deprecated because frozen indices have been deprecated. Consider cold or frozen tiers in place of frozen indices."]
2023-03-08 17:26:00.235 WARN 6936 --- [ main] org.elasticsearch.client.RestClient : request [POST http://192.168.198.129:9200/book_index/_search?typed_keys=true&max_concurrent_shard_requests=5&ignore_unavailable=false&expand_wildcards=open&allow_no_indices=true&ignore_throttled=true&search_type=query_then_fetch&batched_reduce_size=512] returned 2 warnings: [299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "Elasticsearch built-in security features are not enabled. Without authentication, your cluster could be accessible to anyone. See https://www.elastic.co/guide/en/elasticsearch/reference/7.17/security-minimal-setup.html to enable security."],[299 Elasticsearch-7.17.7-78dcaaa8cee33438b91eca7f5c7f56a70fec9e80 "[ignore_throttled] parameter is deprecated because frozen indices have been deprecated. Consider cold or frozen tiers in place of frozen indices."]
Book(id=wnhz001, title=西游记, author=吴承恩, publisher=神话出版社, price=33.5, content=历经千险,终成大道, publishDate=Wed Mar 08 17:25:39 CST 2023)
Process finished with exit code 0
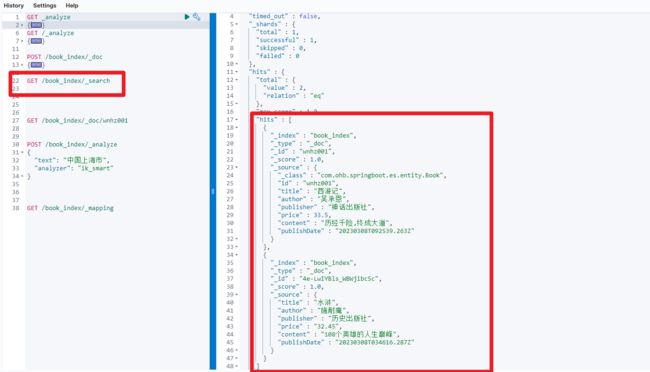
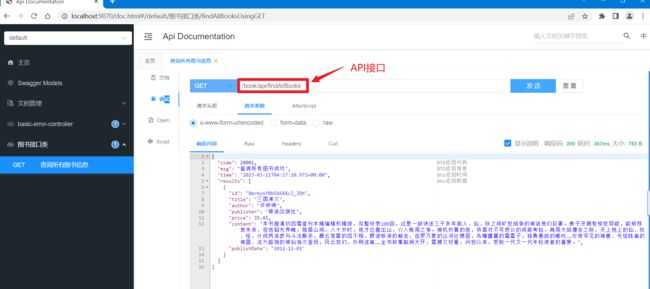