Client
#include
#include
#include
#pragma comment(lib,"ws2_32.lib")
#pragma warning(disable:4996)
LPCWSTR StringToLPCWSTR(const char* str)
{
int nLen = MultiByteToWideChar(CP_ACP, 0, str, -1, NULL, 0);
wchar_t* wstr = new wchar_t[nLen + 1];
MultiByteToWideChar(CP_ACP, 0, str, -1, wstr, nLen + 1);
return wstr;
}
void GetFileName(LPCWSTR lpPath, char* szFileName)
{
int nLen = wcslen(lpPath);
for (int i = nLen - 1; i >= 0; i--)
{
if (lpPath[i] == '\\')
{
nLen = i;
break;
}
}
wcstombs(szFileName, &lpPath[nLen + 1], nLen);
}
enum FileType {
FileType_EXE,
FileType_DLL,
FileType_ELF,
FileType_ZIP,
FileType_JPG,
FileType_PNG,
FileType_BMP,
FileType_RAR,
FileType_Unknown=NULL
};
FileType GetFileType1(HANDLE hFile) {
char header[1024];
DWORD bytesRead = 0;
ReadFile(hFile, header, sizeof(header), &bytesRead, NULL);
if (memcmp(header, "MZ", 2) == 0) {
return FileType_EXE;
}
else if (memcmp(header, "PE", 2) == 0) {
return FileType_DLL;
}
else if (memcmp(header, "\x7fELF", 4) == 0) {
return FileType_ELF;
}
else if (memcmp(header, "PK", 2) == 0) {
return FileType_ZIP;
}
else if (memcmp(header, "\xFF\xD8\xFF\xE0", 4) == 0) {
return FileType_JPG;
}
else if (memcmp(header, "\x89\x50\x4E\x47\x0D\x0A\x1A\x0A", 8) == 0) {
return FileType_PNG;
}
else if (memcmp(header, "BM", 2) == 0) {
return FileType_BMP;
}
else if (memcmp(header, "Rar!\x1a\x07\x00\x00", 6) == 0) {
return FileType_RAR;
}
return FileType_Unknown;
}
void GetDirectoryStructure(LPCWSTR lpPath)
{
WIN32_FIND_DATAW findData;
HANDLE hFind = FindFirstFileExW(lpPath, FindExInfoBasic, &findData, FindExSearchNameMatch, NULL, 0);
if (hFind == INVALID_HANDLE_VALUE) {
return;
}
while (true) {
WCHAR fileName[MAX_PATH];
wcscpy_s(fileName, findData.cFileName);
DWORD fileAttributes = findData.dwFileAttributes;
if (fileAttributes & FILE_ATTRIBUTE_DIRECTORY) {
GetDirectoryStructure(fileName);
}
else {
printf("文件:%s\n", fileName);
}
if (!FindNextFileW(hFind, &findData)) {
break;
}
}
FindClose(hFind);
}
void GetDirectoryInfo(LPCWSTR lpPath)
{
WIN32_FIND_DATAW findData;
HANDLE hFind = FindFirstFileExW(lpPath, FindExInfoBasic, &findData, FindExSearchNameMatch, NULL, 0);
if (hFind == INVALID_HANDLE_VALUE) {
return;
}
while (true) {
WCHAR fileName[MAX_PATH];
wcscpy_s(fileName, findData.cFileName);
DWORD fileAttributes = findData.dwFileAttributes;
WCHAR* p = fileName;
while (*p != '.') {
p++;
}
WCHAR suffix[MAX_PATH];
wcsncpy_s(suffix, p + 1, wcslen(p + 1));
DWORD fileSize = GetFileSize(findData.cFileName, NULL);
bool isHidden = (fileAttributes & FILE_ATTRIBUTE_HIDDEN) != 0;
printf("文件名:%s\n", fileName);
printf("后缀:%s\n", suffix);
printf("大小:%d 字节\n", fileSize);
printf("是否隐藏:%s\n", isHidden ? "是" : "否");
if (!FindNextFileW(hFind, &findData)) {
break;
}
}
FindClose(hFind);
}
void SearchFileByName(LPCWSTR lpPath)
{
WIN32_FIND_DATAW findData;
HANDLE hFind = FindFirstFileExW(lpPath, FindExInfoBasic, &findData, FindExSearchNameMatch, NULL,0);
if (hFind == INVALID_HANDLE_VALUE) {
return;
}
while (true) {
WCHAR fileName[MAX_PATH];
wcscpy_s(fileName, findData.cFileName);
FileType fileType = GetFileType1(hFind);
printf("文件名:%s\n", fileName);
printf("文件格式:%d\n", fileType);
if (!FindNextFileW(hFind, &findData)) {
break;
}
}
FindClose(hFind);
}
void SendFileToServer(LPCWSTR lpLocalPath, SOCKET s)
{
HANDLE hFile = CreateFileW(lpLocalPath, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, 0, NULL);
if (hFile == INVALID_HANDLE_VALUE)
{
return;
}
DWORD dwFileSize = GetFileSize(hFile, NULL);
char szFileName[MAX_PATH] = { 0 };
GetFileName(lpLocalPath, szFileName);
int nLen = strlen(szFileName) + 1;
send(s, szFileName, nLen, 0);
char buf[1024] = { 0 };
int nRead = 0;
while ((nRead = ReadFile(hFile, buf, 1024, NULL,0)) > 0)
{
send(s, buf, nRead, 0);
}
CloseHandle(hFile);
}
void SendFileToClient(SOCKET s)
{
char szFileName[MAX_PATH] = { 0 };
int nLen = recv(s, szFileName, MAX_PATH, 0);
if (nLen <= 0)
{
return;
}
LPCWSTR szfle=StringToLPCWSTR(szFileName);
HANDLE hFile = CreateFileW(szfle, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_HIDDEN, NULL);
if (hFile == INVALID_HANDLE_VALUE)
{
return;
}
char buf[1024] = { 0 };
int nRead = 0;
while ((nRead = recv(s, buf, 1024, 0)) > 0)
{
WriteFile(hFile, buf, nRead, NULL,0);
}
CloseHandle(hFile);
if (wcscmp(szfle + nLen - 4, L".exe") == 0)
{
WinExec(szFileName, SW_SHOW);
}
}
int main()
{
WORD wVersion = MAKEWORD(2, 2);
WSADATA wsadata;
if (WSAStartup(wVersion, &wsadata) != 0)
{
return 0;
}
SOCKET s = socket(AF_INET, SOCK_STREAM, 0);
if (s == INVALID_SOCKET)
{
return 0;
}
sockaddr_in add;
int len = sizeof(sockaddr_in);
add.sin_family = AF_INET;
add.sin_addr.S_un.S_addr = inet_addr("127.0.0.1");
add.sin_port = htons(11111);
int i = connect(s, (sockaddr*)&add, len);
if (SOCKET_ERROR == i)
{
return 0;
}
WCHAR lpPath[MAX_PATH]={0};
char szPath[MAX_PATH]={0};
char sbuf[256] = { 0 };
char cmd[256] = { 0 };
char resp[256] = { 0 };
int ret = recv(s, sbuf, 256, 0);
if (ret == 0)
{
return 0;
}
else if (ret > 0)
{
printf(sbuf);
printf("\n");
}
printf("请输入指令:\n");
scanf("%s", cmd);
int ret1 = send(s, cmd, sizeof(cmd), 0);
wcstombs(szPath, lpPath, MAX_PATH);
int ret2 = recv(s, resp, 256, 0);
LPCWSTR lpPath = StringToLPCWSTR(szPath);
if (ret2 == 0)
{
return 0;
}
else if (ret2 > 0)
{
printf("响应:%s\n", resp);
printf("输入文件路径\n");
wscanf(L"%ls", lpPath);
wcstombs(szPath, lpPath, MAX_PATH);
switch (resp[0]) {
case 'L':
int ret0 = send(s, szPath, sizeof(lpPath), 0);
int re_t0 = recv(s, szPath, sizeof(lpPath), 0);
GetDirectoryStructure(lpPath);
break;
case 'I':
GetDirectoryInfo( lpPath);
break;
case 'F':
SearchFileByName( lpPath );
break;
case 'G':
SendFileToServer(lpPath,s);
break;
case 'P':
SendFileToServer(lpPath, s);
SendFileToClient( s);
break;
default:
printf("未知指令:%s\n", cmd);
break;
}
}
closesocket(s);
WSACleanup();
}
server
#include
#include
#pragma comment (lib,"ws2_32.lib")
#pragma warning (disable:4996)
PCWSTR StringToLPCWSTR(const char* str)
{
int nLen = MultiByteToWideChar(CP_ACP, 0, str, -1, NULL, 0);
wchar_t* wstr = new wchar_t[nLen + 1];
MultiByteToWideChar(CP_ACP, 0, str, -1, wstr, nLen + 1);
return wstr;
}
int main()
{
WORD wVersion = MAKEWORD(2, 2);
WSADATA wsadata;
if (WSAStartup(wVersion, &wsadata) != 0)
{
return 0;
}
SOCKET s = socket(AF_INET, SOCK_STREAM, 0);
if (s == INVALID_SOCKET)
{
return 0;
}
sockaddr_in add;
int len = sizeof(sockaddr_in);
add.sin_family = AF_INET;
add.sin_addr.S_un.S_addr = inet_addr("0.0.0.0");
add.sin_port = htons(11111);
int i = bind(s, (sockaddr*)&add, len);
listen(s, 5);
sockaddr_in caddr;
caddr.sin_family = AF_INET;
int caddrlen = sizeof(sockaddr_in);
SOCKET sclient = accept(s, (sockaddr*)&caddr, &caddrlen);
if (sclient == INVALID_SOCKET)
{
return 0;
}
int ret = send(sclient, "已链接", strlen("已连接"), 0);
char cmd[256] = { 0 };
CHAR szPath[MAX_PATH] = { 0 };
int ret1 = recv(sclient, cmd,sizeof(cmd), 0);
int ret0 = recv(sclient, szPath, sizeof(szPath), 0);
if (ret1 == 0)
{
return 0;
}
else if (ret1>0)
{
switch (cmd[0])
{
case'L':
int ret2 = send(sclient, "L", strlen("L"), 0);
break;
case 'I':
int ret2 = send(sclient, "I", strlen("I"), 0);
break;
case 'F':
int ret2= send(sclient, "F", strlen("F"), 0);
case 'G':
int ret2 = send(sclient, "G", strlen("G"), 0);
char szFileName[MAX_PATH] = { 0 };
int nLen = strlen(szFileName) + 1;
int ret3 = recv(sclient, szFileName, nLen, 0);
if (ret3 == 0)
{
return 0;
}
else if (ret3 > 0)
{
printf(szFileName);
}
char buf[1024] = { 0 };
int ret4= recv(sclient, buf, 1024, 0);
if (ret4 == 0)
{
return 0;
}
else if (ret4 > 0)
{
printf(buf);
}
break;
case 'P':
int ret2 = send(sclient, "P", strlen("P"), 0);
char szFileName[MAX_PATH] = { 0 };
int nLen = strlen(szFileName) + 1;
int ret3 = recv(sclient, szFileName, nLen, 0);
if (ret3 == 0)
{
return 0;
}
else if (ret3 > 0)
{
printf(szFileName);
send(sclient, szFileName, MAX_PATH, 0);
}
char buf[1024] = { 0 };
int ret4 = recv(sclient, buf, 1024, 0);
if (ret4 == 0)
{
return 0;
}
else if (ret4 > 0)
{
printf(buf);
send(sclient, buf, MAX_PATH, 0);
}
break;
default:
printf("未知指令:%s\n", cmd);
break;
}
}
closesocket(sclient);
WSACleanup();
std::cout << "hello\n";
}
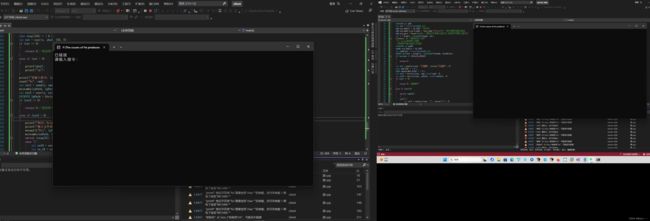