文章目录
- 1 车辆统计项目
-
- 2 特征点检测和匹配
-
- 2.1 harris角点检测
- 2.2 shi-tomasi角点检测
- 2.3 SIFT关键点检测
- 2.4 SURF特征检测
- 2.5 ORB特征检测
- 3 特征匹配
-
- 3.1 暴力特征匹配
- 3.2 FLANN特征匹配
- 3.3 图像查找
-
- 4 模版匹配
-
- 4.1 模版匹配
- 4.2 匹配多个对象
- 4.3 处理模版图片
- 4.4 数字模版处理和信用卡图片形态学操作
- 5 图像分割
-
- 5.1 分水岭法
- 5.2 GrabCut
- 5.3 MeanShift
- 6 图像修复
- 7 人脸检测
1 车辆统计项目
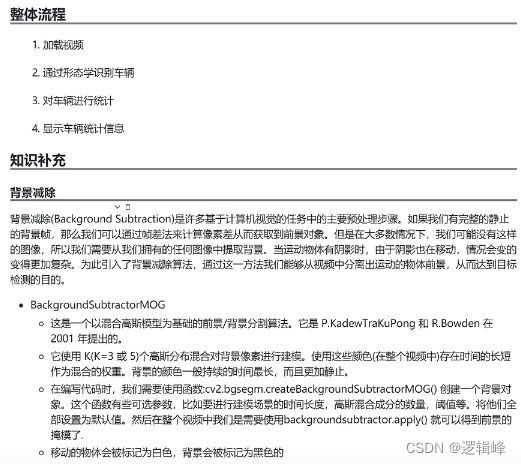
import cv2
import numpy as np
cap = cv2.VideoCapture('./video/car.mp4')
while True:
ret, frame = cap.read()
if ret:
cv2.imshow('video',frame)
key = cv2.waitKey(1)
if key == 27:
break
cap.release()
cv2.destroyAllWindows()
1.1 背景减除
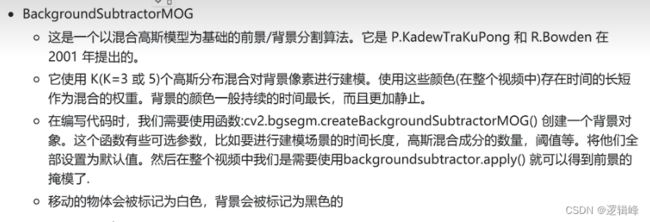
import cv2
import numpy as np
cap = cv2.VideoCapture(0)
bgs = cv2.bgsegm.createBackgroundSubtractorMOG()
while True:
ret, frame = cap.read()
if ret:
fgmask = bgs.apply(frame)
cv2.imshow('video', fgmask)
key = cv2.waitKey(1)
if key == 27:
break
cap.release()
cv2.destroyAllWindows()
1.2 车辆统计
import cv2
import numpy as np
cap = cv2.VideoCapture('./video/car.mp4')
mog = cv2.bgsegm.createBackgroundSubtractorMOG()
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
min_w = 40
min_h = 30
line_high = 630
cars = []
offset = 6
car_num = 0
def center(x, y, w, h):
x1 = int(w / 2)
y1 = int(h / 2)
cx = int(x) + x1
cy = int(y) + y1
return cx, cy
while True:
ret, frame = cap.read()
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_RGB2GRAY)
blur = cv2.GaussianBlur(gray, (3, 3), 5)
mask = mog.apply(blur)
erode = cv2.erode(mask, kernel, iterations=2)
dialte = cv2.dilate(erode, kernel, iterations=2)
close = cv2.morphologyEx(dialte, cv2.MORPH_CLOSE, kernel)
cv2.line(frame, [10, line_high], [1270, line_high], [255, 0, 0], 2)
contours, h = cv2.findContours(close, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
is_valid = (w >= min_w) and (h >= min_h)
if not is_valid:
continue
cv2.rectangle(frame, (int(x), int(y)), (int(x + w), int(y + h)), (0, 0, 255), 2)
cpoint = center(x, y, w, h)
cars.append(cpoint)
cv2.circle(frame, (cpoint), 5, (0, 0, 255), -1)
for (x, y) in cars:
if (line_high - offset) < y < (line_high + offset):
car_num += 1
cars.remove((x, y))
print(car_num)
cv2.putText(frame, 'Vechicle Count:' + str(car_num), [500, 60], cv2.FONT_HERSHEY_SIMPLEX, 2, [0, 0, 255], 5)
cv2.imshow('frame', frame)
key = cv2.waitKey(3)
if key == 27:
break
cap.release()
cv2.destroyAllWindows()
2 特征点检测和匹配
2.1 harris角点检测
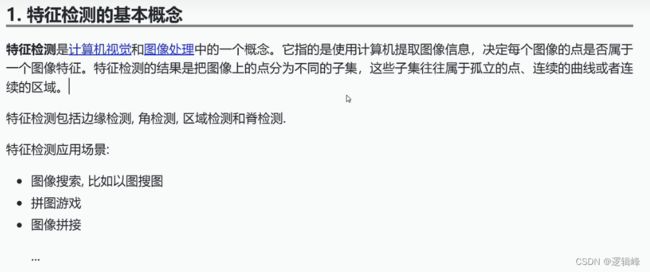
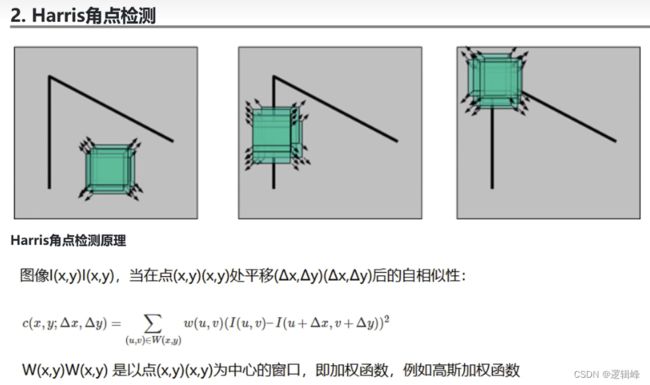
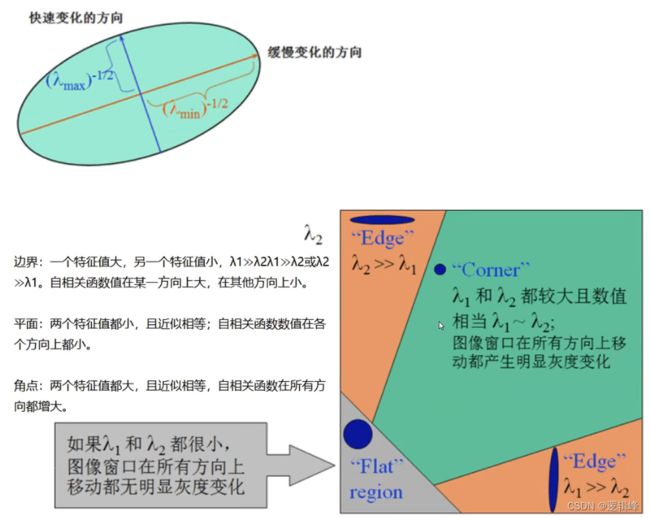
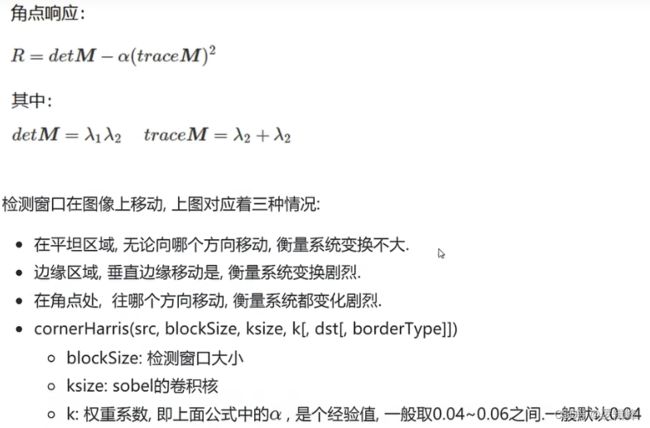
import cv2
import numpy as np
img = cv2.imread('./image/dog.png')
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
dst = cv2.cornerHarris(gray, blockSize=2, ksize=3, k=0.04)
print(dst)
print(dst.shape)
img[dst > 0.01* dst.max()]= [0,0,255]
cv2.imshow('img',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
2.2 shi-tomasi角点检测
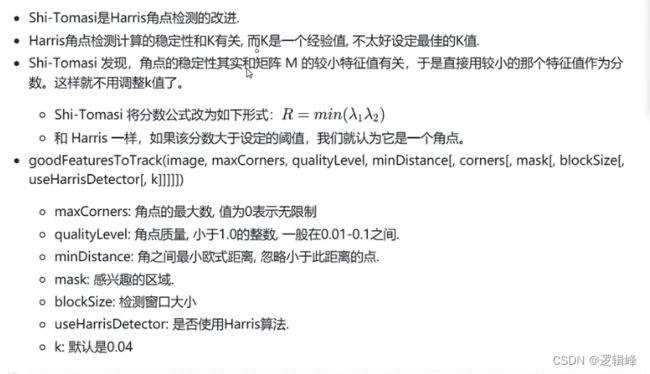
import cv2
import numpy as np
maxCorners = 1000
ql = 0.01
minDistance = 10
img = cv2.imread('./image/dog.png')
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
corners = cv2.goodFeaturesToTrack(gray,maxCorners,ql, minDistance)
corners = np.int16(corners)
for i in corners:
x,y = i.ravel()
cv2.circle(img,(x,y), 3,(255,0,0