1、题目:
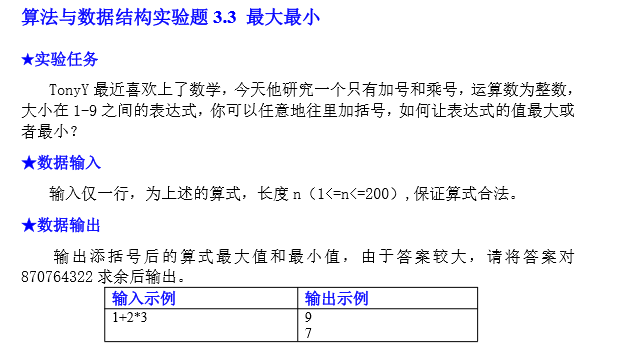
2、代码:
#include
#include
#include
typedef struct astack *Stack;
typedef struct astack
{
__int64 top;
__int64 *data;
} Astack;
typedef struct stack *Operator;
typedef struct stack
{
__int64 topOperator;
char *ch;
} sta;
void PushNumber(Stack S,__int64 x)
{
S->data[++S->top]=x%870764322;
}
void PushOperator(Operator o,char c)
{
o->ch[++o->topOperator]=c;
}
int StackEmpty(Stack S)
{
return S->top<0;
}
int OperatorEmpty(Operator o)
{
return o->topOperator<0;
}
char PopOperator(Operator o)
{
return o->ch[o->topOperator--];
}
int PopNumber(Stack S)
{
return S->data[S->top--]%870764322;
}
int main()
{
char str[201];
gets(str);
int length=strlen(str);
Stack S=(Stack)malloc(sizeof*S);
S->data=(__int64*)malloc(length*sizeof(__int64));
S->top=-1;
Operator o=(Operator)malloc(sizeof*o);
o->ch=(char*)malloc(length*sizeof(char));
o->topOperator=-1;
int i;
__int64 a1,a2,sum,max,min;
//计算最小值,先算乘法
while (!StackEmpty(S)) PopNumber(S);
while (!OperatorEmpty(o)) PopOperator(o);
for(i=0; i='0'&&str[i]<='9')
{
PushNumber(S,str[i]-48);
}
else
{
if(OperatorEmpty(o))
{
PushOperator(o,str[i]);
}
else
{
while(str[i] == '+' && o->ch[o->topOperator]== '*')
{
a1=(__int64)S->data[S->top]%870764322;
PopNumber(S);
a2=(__int64)S->data[S->top]%870764322;
PopNumber(S);
sum=(a1*a2)%870764322;
PushNumber(S,sum);
PopOperator(o);
if(OperatorEmpty(o))
{
break;
}
}
PushOperator(o,str[i]);
}
}
}
while(!OperatorEmpty(o))
{
a1=(__int64)S->data[S->top]%870764322;
PopNumber(S);
a2=(__int64)S->data[S->top]%870764322;
PopNumber(S);
if(o->ch[o->topOperator]=='+')
{
sum=(a1+a2)%870764322;
}
else
{
sum=(a1*a2)%870764322;
}
PushNumber(S,sum);
PopOperator(o);
}
min=S->data[S->top];
//计算最大值,先做加法运算
//存数字和符号的栈清空,重新存
while (!StackEmpty(S)) PopNumber(S);
while (!OperatorEmpty(o)) PopOperator(o);
for(i=0; i='0'&&str[i]<='9')
{
PushNumber(S,str[i]-48);
}
else
{
if(OperatorEmpty(o))
{
PushOperator(o,str[i]);
}
else
{
while(str[i] == '*' && o->ch[o->topOperator]== '+')
{
a1=(__int64)S->data[S->top]%870764322;
PopNumber(S);
a2=(__int64)S->data[S->top]%870764322;
PopNumber(S);
sum=(a1+a2)%870764322;
PushNumber(S,sum);
PopOperator(o);
if(OperatorEmpty(o))
{
break;
}
}
PushOperator(o,str[i]);
}
}
}
while(!OperatorEmpty(o))
{
a1=(__int64)S->data[S->top]%870764322;
PopNumber(S);
a2=(__int64)S->data[S->top]%870764322;
PopNumber(S);
if(o->ch[o->topOperator]=='*')
{
sum=(a1*a2)%870764322;
}
else
{
sum=(a1+a2)%870764322;
}
PushNumber(S,sum);
PopOperator(o);
}
max=S->data[S->top];
printf("%d\n",max);
printf("%d\n",min);
return 0;
}
3、大神代码:
#include
#include
#include
#include
#include
#include
#include