1.依赖
com.itextpdf
itextpdf
5.5.10
com.itextpdf
itext-asian
5.2.0
org.bouncycastle
bcprov-jdk15on
1.65
2.工具类
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.*;
import lombok.SneakyThrows;
import java.io.IOException;
public class PdfPageSongTiUtil extends PdfPageEventHelper {
/**
* 文档字体大小,页脚页眉最好和文本大小一致
*/
public int presentFontSize = 10;
/**
* 文档页面大小,最好前面传入,否则默认为A4纸张
*/
public Rectangle pageSize = PageSize.A4;
// 模板
public PdfTemplate total;
// 基础字体对象
public BaseFont bf = null;
// 利用基础字体生成的字体对象,一般用于生成中文文字
public Font fontDetail = null;
/**
*
* 无参构造方法.
*
*/
public PdfPageSongTiUtil() {
}
public PdfPageSongTiUtil(int presentFontSize, Rectangle pageSize) {
this.presentFontSize = presentFontSize;
this.pageSize = pageSize;
}
public void setPresentFontSize(int presentFontSize) {
this.presentFontSize = presentFontSize;
}
/**
*
* 文档打开时创建模板
*/
@Override
public void onOpenDocument(PdfWriter writer, Document document) {
// 共 页 的矩形的长宽高
total = writer.getDirectContent().createTemplate(50, 50);
}
/**
*
*关闭每页的时候,写入页眉
*/
@SneakyThrows
@Override
public void onEndPage(PdfWriter writer, Document document) {
this.addPage(writer, document);
}
//加分页
public void addPage(PdfWriter writer, Document document) throws IOException, DocumentException {
//设置分页页眉页脚字体
try {
if (bf == null) {
bf = BaseFont.createFont("template/GB2312.ttf", BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
}
if (fontDetail == null) {
fontDetail = new Font(bf, presentFontSize, Font.NORMAL);// 数据体字体
}
} catch (DocumentException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
// 1.写入页眉
// ColumnText.showTextAligned(writer.getDirectContent(),
// Element.ALIGN_LEFT, new Phrase(header, fontDetail),
// document.left(), document.top() + 20, 0);
// 2.写入前半部分的 第 X页/共
Phrase footer = new Phrase("来源:中学生统一服务平台", fontDetail);
// 4.拿到当前的PdfContentByte
PdfContentByte cb = writer.getDirectContent();
// 5.写入页脚1,x轴就是(右margin+左margin + right() -left()- len)/2.0F
ColumnText
.showTextAligned(
cb,
Element.ALIGN_CENTER,
footer,
document.right()-document.rightMargin()-5 ,
document.bottom() - 10, 0);
// 调节模版显示的位置
//加水印
addWatermark(writer);
}
/**
*
* 关闭文档时,替换模板,完成整个页眉页脚组件
*/
@Override
public void onCloseDocument(PdfWriter writer, Document document) {
// 关闭文档的时候,将模板替换成实际的 Y 值
total.beginText();
// 生成的模版的字体、颜色
total.setFontAndSize(bf, presentFontSize);
//页脚内容拼接 如 第1页/共2页
//String foot2 = " " + (writer.getPageNumber()) + " 页";
//页脚内容拼接 如 第1页/共2页
String foot2 = String.valueOf(writer.getPageNumber());
// 模版显示的内容
total.showText(foot2);
total.endText();
total.closePath();
}
// 加水印
public void addWatermark(PdfWriter writer) throws IOException, DocumentException {
PdfContentByte waterMar = writer.getDirectContentUnder();
String text="全国中学生会议";
waterMar.beginText();
PdfGState gs=new PdfGState();
//透明度
gs.setFillOpacity(0.2F);
waterMar.setFontAndSize(BaseFont.createFont("template/GB2312.ttf", BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED),12);
waterMar.setGState(gs);
for (int x = 0; x <=800; x+=200) {
for (int y = 0; y < 800; y+=100) {
//水印对齐方式 水印内容 x坐标 y坐标 旋转角度
waterMar.showTextAligned(Element.ALIGN_RIGHT,text,x,y,35);
}
}
waterMar.setColorFill(BaseColor.GRAY);
waterMar.endText();
waterMar.stroke();
}
}
3.实体类
@Data
public class PersonnelInfo {
private String name;
private String phoneNumber;
private String sex;
private String schoolName;
private String workerPosition;
private String workerDate;
private String certificate;
private String OtherCertificate;
}
4. 例子
@GetMapping(value = "/createFilePdf", produces = MediaType.APPLICATION_JSON_VALUE)
public void createFilePdf(HttpServletRequest request, HttpServletResponse response) throws IOException, DocumentException {
request.getSession();
response.setContentType("application/pdf;charset=UTF-8");
response.setCharacterEncoding("utf-8");
String fileName =URLEncoder.encode( "导出pdf人员登记表","UTF-8");
response.setHeader("Content-Disposition", "attachment;filename*=utf-8''" + fileName+".pdf");
List list=new ArrayList<>();
PersonnelInfo personnel=new PersonnelInfo();
personnel.setName("张三");
personnel.setSex("男");
personnel.setPhoneNumber("1101001001");
personnel.setSchoolName("清华附中");
personnel.setWorkerPosition("北京");
personnel.setWorkerDate("1999-12-12");
personnel.setCertificate("特级数学老师");
personnel.setOtherCertificate("二级心理咨询");
list.add(personnel);
// 定义全局的字体静态变量
Font content = null;
Font fontHead = null;
try {
// 不同字体(这里定义同一种字体:包含不同字号、不同style)
BaseFont bfChinese = BaseFont.createFont("template/GB2312.ttf", BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
content = new Font(bfChinese, 11, Font.NORMAL);
//使用字体并给出颜色
fontHead = new Font(bfChinese,20,Font.BOLD,BaseColor.BLACK);
} catch (Exception e) {
e.printStackTrace();
}
Document document=new Document(new RectangleReadOnly(850F,590F));
document.setMargins(50,50,45,45);
PdfWriter writer = PdfWriter.getInstance(document, response.getOutputStream());
//用户密码 保证不能修改 设置所有者密码即可
String pwdUser="";
//所有者密码
String pwdOwn="18956723.";
writer.setEncryption("".getBytes(),pwdOwn.getBytes(),PdfWriter.ALLOW_PRINTING,PdfWriter.ENCRYPTION_AES_128);
//添加页脚、水印等
PdfPageSongTiUtil myHeadFooter=new PdfPageSongTiUtil();
writer.setPageEvent(myHeadFooter);
//open
document.open();
Paragraph paragraphHead1=new Paragraph("人员信息列表",fontHead);
paragraphHead1.setAlignment(Element.ALIGN_CENTER);
document.add(paragraphHead1);
document.add(new Paragraph("\n"));
PdfPCell cellBg[]=new PdfPCell[2];
float[] width={35f,30f};
//创建表格
PdfPTable table=new PdfPTable(width);
table.setWidthPercentage(100.0f);
//表格顶端文本
PdfPCell celltTableTop[]=new PdfPCell[2];
float[] widthTop={55f,15f};
PdfPTable tableTop=new PdfPTable(widthTop);
tableTop.setWidthPercentage(100.0f);
celltTableTop[0]=new PdfPCell(new Paragraph("会议开班负责人(签字):",content));
celltTableTop[0].setBorder(0);
tableTop.addCell(celltTableTop[0]);
celltTableTop[1]=new PdfPCell(new Paragraph("日期:",content));
celltTableTop[1].setBorder(0);
tableTop.addCell(celltTableTop[1]);
document.add(tableTop);
//数据列
PdfPCell cell=null;
//11列 人员表头
float[] width2={10f,15f,10f,20f,25f,25f,25f,25f,25f};
PdfPTable tabl2=new PdfPTable(width2);
PdfPTableHeader pdfPTableHeader=new PdfPTableHeader();
tabl2.setSpacingBefore(5f);
tabl2.setWidthPercentage(100.0f);
//表头 换页显示
tabl2.setHeaderRows(1);
tabl2.getDefaultCell().setHorizontalAlignment(1);
List listTitle=Arrays.asList("序号","姓名","性别","联系方式","工作地点","工作岗位","入职日期","教学相关资格证书","其他相关资质证书");
for (String title : listTitle) {
tabl2.addCell(createCell(title,content));
}
int index=0;
for (PersonnelInfo personnelInfo : list) {
index++;
PdfPCell cel1=new PdfPCell(new Paragraph(String.valueOf(index),content));
PdfPCell cel2=new PdfPCell(new Paragraph(personnelInfo.getName(),content));
PdfPCell cel3=new PdfPCell(new Paragraph(personnelInfo.getSex(),content));
PdfPCell cel4=new PdfPCell(new Paragraph(personnelInfo.getPhoneNumber(),content));
PdfPCell cel5=new PdfPCell(new Paragraph(personnelInfo.getSchoolName(),content));
PdfPCell cel6=new PdfPCell(new Paragraph(personnelInfo.getWorkerPosition(),content));
PdfPCell cel7=new PdfPCell(new Paragraph(personnelInfo.getWorkerDate(),content));
PdfPCell cel8=new PdfPCell(new Paragraph(personnelInfo.getCertificate(),content));
PdfPCell cel9=new PdfPCell(new Paragraph(personnelInfo.getOtherCertificate(),content));
cel1.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel1.setHorizontalAlignment(Element.ALIGN_CENTER);
cel2.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel2.setHorizontalAlignment(Element.ALIGN_CENTER);
cel3.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel3.setHorizontalAlignment(Element.ALIGN_CENTER);
cel4.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel4.setHorizontalAlignment(Element.ALIGN_CENTER);
cel5.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel5.setHorizontalAlignment(Element.ALIGN_CENTER);
cel6.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel6.setHorizontalAlignment(Element.ALIGN_CENTER);
cel7.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel7.setHorizontalAlignment(Element.ALIGN_CENTER);
cel8.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel8.setHorizontalAlignment(Element.ALIGN_CENTER);
cel9.setVerticalAlignment(Element.ALIGN_MIDDLE);
cel9.setHorizontalAlignment(Element.ALIGN_CENTER);
tabl2.addCell(cel1);
tabl2.addCell(cel2);
tabl2.addCell(cel3);
tabl2.addCell(cel4);
tabl2.addCell(cel5);
tabl2.addCell(cel6);
tabl2.addCell(cel7);
tabl2.addCell(cel8);
tabl2.addCell(cel9);
}
document.add(tabl2);
Paragraph paragraphEnd1=new Paragraph("会议结束负责人签字:",content);
paragraphEnd1.setIndentationLeft(460);
paragraphEnd1.setSpacingBefore(10f);
document.add(paragraphEnd1);
//close
document.close();
}
/**
*
* @param cont 表头
* @param font 字体
* @return
*/
static PdfPCell createCell(String cont,Font font){
PdfPCell cell=new PdfPCell(new Paragraph(cont,font));
cell.setVerticalAlignment(Element.ALIGN_MIDDLE);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
cell.setFixedHeight(30);
cell.setBackgroundColor(new BaseColor(153,203,255));
return cell;
}
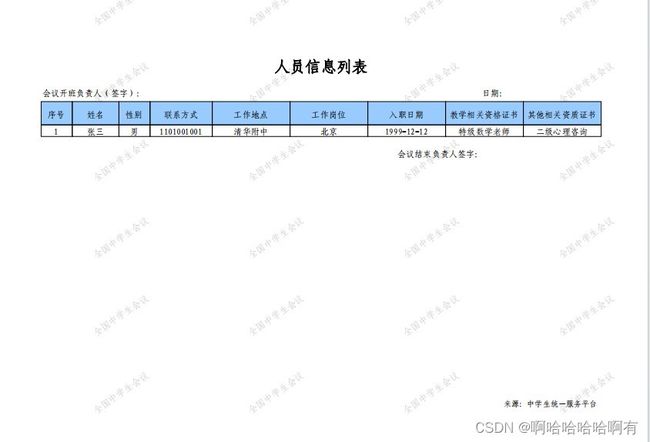