解决散列冲突
-
- 文件结构
- 字典类
-
- 哈希类
-
- 有序链表
-
- 哈希表实现
-
- 测试主函数
-
文件结构

字典类
概念
代码
template<class K,class E>
class dictionary
{
public:
virtual ~dictionary(){}
virtual bool empty() const = 0;
virtual int size() const = 0;
virtual std::pair<const K, E>* find(const K& theKey)const = 0;
virtual void erase(const K& theKey) = 0;
virtual void insert(const std::pair<const K, E>& thePair) = 0;
};
哈希类
概念
代码
#pragma once
#include
template<class K> class hash;
template<>
class hash<std::string>
{
public:
std::size_t operator() (const std::string theKey) const{
unsigned long hashValue = 0;
int length = (int)theKey.length();
for(int i = 0; i < length; i++){
hashValue = hashValue * 5 + theKey.at(i);
}
return std::size_t(hashValue);
}
};
template<>
class hash<int>
{
public:
std::size_t operator() (const int theKey) const{
return std::size_t(theKey);
}
};
template<>
class hash<long>
{
public:
std::size_t operator() (const long theKey) const{
return std::size_t(theKey);
}
};
有序链表
概念
代码
#pragma once
#include
template<class K, class E>
struct pairNode{
std::pair<const K, E> element;
pairNode<K, E>* next;
pairNode(const std::pair<const K, E>& thePair)
:element(thePair) {}
pairNode(const std::pair<const K, E>& thePair, pairNode<K, E>* theNext)
:element(thePair) { this->next = theNext; }
};
template<class K, class E>
class sortedChain
{
private:
pairNode<K,E>* firstNode;
int dSize;
public:
sortedChain();
~sortedChain();
bool isEmpty() const;
int size() const;
std::pair<const K, E>* find(const K& theKey) const;
void insert(const std::pair<const K, E>& thePair);
void erase(const K& theKey);
void printChain(std::ostream &out) const;
};
template<class K, class E>
sortedChain<K, E>::sortedChain(){
this->firstNode = nullptr;
this->dSize = 0;
}
template<class K, class E>
sortedChain<K, E>::~sortedChain(){
pairNode<K, E>* tempNode;
while(this->firstNode){
tempNode = this->firstNode->next;
delete this->firstNode;
this->firstNode = tempNode;
}
}
template<class K, class E>
bool sortedChain<K, E>::isEmpty() const{
return this->dSize == 0;
};
template<class K, class E>
int sortedChain<K, E>::size() const{
return this->dSize;
};
template<class K, class E>
std::pair<const K, E>* sortedChain<K, E>::find(const K& theKey) const{
pairNode<K, E>* tempNode = firstNode;
while((tempNode != nullptr)
&& (tempNode->element.first != theKey)){
tempNode = tempNode->next;
}
if((tempNode != nullptr)
&& (tempNode->element.first == theKey)){
return &tempNode->element;
}
return nullptr;
};
template<class K, class E>
void sortedChain<K, E>::insert(const std::pair<const K, E>& thePair){
pairNode<K, E>* afterInsertNode = this->firstNode;
pairNode<K, E>* beforeInsertNode = nullptr;
while((afterInsertNode != nullptr)
&& (afterInsertNode->element.first < thePair.first)){
beforeInsertNode = afterInsertNode;
afterInsertNode = afterInsertNode->next;
}
if((afterInsertNode != nullptr)
&& (afterInsertNode->element.first == thePair.first)){
afterInsertNode->element.second = thePair.second;
return;
}
pairNode<K, E>* insertNode = new pairNode<K, E>(thePair, afterInsertNode);
if(beforeInsertNode == nullptr){
this->firstNode = insertNode;
}else{
beforeInsertNode->next = insertNode;
}
this->dSize++;
};
template<class K, class E>
void sortedChain<K, E>::erase(const K& theKey){
pairNode<K, E>* eraseNode = this->firstNode;
pairNode<K, E>* beforeEraseNode = nullptr;
while((eraseNode != nullptr)
&&(eraseNode->element.first < theKey)){
beforeEraseNode = eraseNode;
eraseNode = eraseNode->next;
}
if((eraseNode != nullptr)
&& (eraseNode->element.first == theKey)){
if(beforeEraseNode == nullptr){
this->firstNode = eraseNode->next;
}else{
beforeEraseNode->next = eraseNode->next;
}
delete eraseNode;
this->dSize--;
}
};
template<class K, class E>
void sortedChain<K, E>::printChain(std::ostream& out) const{
for(pairNode<K, E>* tempNode = this->firstNode; tempNode != nullptr; tempNode = tempNode->next){
out << "key: " << tempNode->element.first;
out << ", value: " << tempNode->element.second;
out << " ";
}
out << std::endl;
};
template<class K,class E>
std::ostream& operator<<(std::ostream& out, const sortedChain<K, E>& theChain){
theChain.printChain(out);
return out;
}
哈希表实现
概念
代码
#pragma once
#include "dictionary.h"
#include "hash.h"
#include "sortedChain.h"
template<class K, class E>
class hashChains : public dictionary<K, E>
{
private:
sortedChain<K, E>* table;
hash<K> myHash;
int dSize;
int divisor = 11;
public:
hashChains(int theDivisor);
~hashChains();
bool empty() const override;
int size() const override;
std::pair<const K, E>* find(const K& theKey)const override;
void erase(const K& theKey) override;
void insert(const std::pair<const K, E>& thePair) override;
void printHashChain(std::ostream& out) const;
};
template<class K, class E>
hashChains<K, E>::hashChains(int theDivisor){
this->divisor = theDivisor;
this->dSize = 0;
this->table = new sortedChain<K, E>[theDivisor];
}
template<class K, class E>
hashChains<K, E>::~hashChains(){
if(this->table){
delete[] this->table;
}
}
template<class K, class E>
bool hashChains<K, E>::empty() const{
return (this->dSize == 0);
}
template<class K, class E>
int hashChains<K, E>::size() const{
return this->dSize;
}
template<class K, class E>
std::pair<const K, E>* hashChains<K, E>::find(const K& theKey)const{
int bucketIndex = myHash(theKey) % this->divisor;
return this->table[bucketIndex].find(theKey);
}
template<class K, class E>
void hashChains<K, E>::erase(const K& theKey){
int bucketIndex = myHash(theKey) % this->divisor;
return this->table[bucketIndex].erase(theKey);
}
template<class K, class E>
void hashChains<K, E>::insert(const std::pair<const K, E>& thePair){
int bucketIndex = myHash(thePair.first) % this->divisor;
if(!this->table[bucketIndex].find(thePair.first)){
this->dSize++;
}
this->table[bucketIndex].insert(thePair);
}
template<class K, class E>
void hashChains<K, E>::printHashChain(std::ostream& out) const{
for(int i = 0; i < this->divisor; ++i){
if(this->table[i].isEmpty()){
std::cout << "NULL" << std::endl;
}else{
std::cout << this->table[i];
}
}
}
template<class K, class E>
std::ostream& operator<<(std::ostream& out, const hashChains<K, E>& theHashChains){
theHashChains.printHashChain(out);
return out;
}
测试主函数
代码
#include
#include
#include "hashChains.h"
using namespace std;
int main(){
hashChains<string, int> myHashChains1(11);
myHashChains1.insert(pair<string, int>("abc", 2));
myHashChains1.insert(pair<string, int>("def", 3));
myHashChains1.insert(pair<string, int>("ghi", 4));
myHashChains1.insert(pair<string, int>("jkl", 5));
myHashChains1.insert(pair<string, int>("mno", 6));
myHashChains1.insert(pair<string, int>("pqrs", 7));
myHashChains1.insert(pair<string, int>("tuv", 8));
myHashChains1.insert(pair<string, int>("wxyz", 9));
hashChains<int, int> myHashChains2(11);
myHashChains2.insert(pair<int, int>(1, 1));
myHashChains2.insert(pair<int, int>(2, 2));
myHashChains2.insert(pair<int, int>(3, 3));
myHashChains2.insert(pair<int, int>(5, 5));
myHashChains2.insert(pair<int, int>(11, 11));
myHashChains2.insert(pair<int, int>(13, 13));
myHashChains2.insert(pair<int, int>(10, 10));
cout << myHashChains1 << endl << endl;
cout << myHashChains2 << endl;
return 0;
}
输出
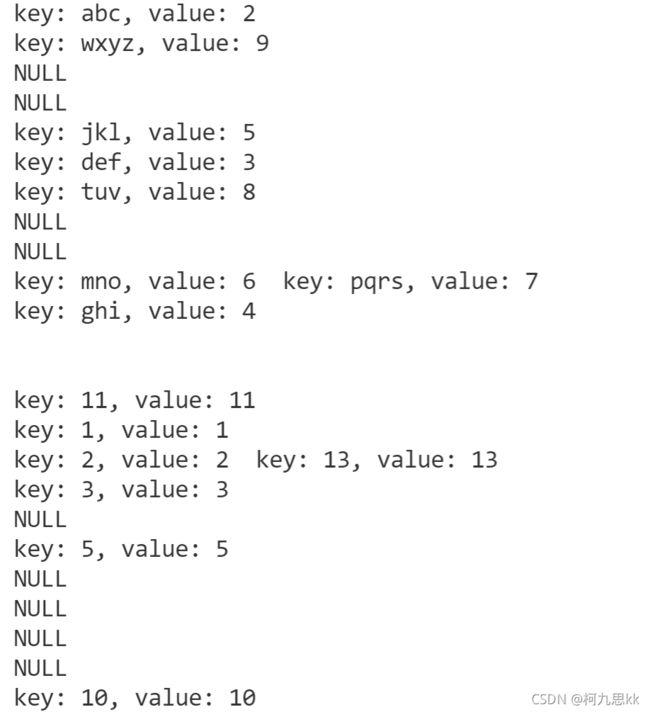
一个sortedChain在同一行显示。