文章目录
- 断言式自定义异常
-
-
- 枚举异常接口限制
- 自定义异常
- 断言方式抛出异常
- 枚举类
- 使用
- 在web中全局捕捉
断言式自定义异常
枚举异常接口限制
public interface ExEnum {
Integer getCode();
String getMessage();
}
自定义异常
public class DlpException extends RuntimeException {
private static final long serialVersionUID = 1L;
protected Integer errCode;
private Object data;
public Integer getErrCode() {
return errCode;
}
public DlpException(ExEnum exceptionEnum) {
super(exceptionEnum.getMessage());
this.errCode = exceptionEnum.getCode();
}
public DlpException(ExEnum exceptionEnum, String message) {
super(message);
this.errCode = exceptionEnum.getCode();
}
public DlpException(ExEnum exceptionEnum, String message, Object data) {
super(message);
this.data = data;
this.errCode = exceptionEnum.getCode();
}
@Deprecated
public DlpException(String errMsg) {
super(errMsg);
this.errCode = DlpCodeEnum.FAIL.getCode();
}
public DlpException(Throwable e) {
super(e);
}
@Deprecated
public DlpException(String errMsg, Throwable e) {
super(errMsg, e);
this.errCode = DlpCodeEnum.FAIL.getCode();
}
public DlpException(Integer errorCode, String errMsg) {
super(errMsg);
this.errCode = errorCode;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
@Override
public Throwable fillInStackTrace() {
return this;
}
}
断言方式抛出异常
import java.util.Collection;
import java.util.Objects;
public enum DlpAssert {
INSTANT;
private DlpCodeEnum ee;
private String msg;
private Object data;
public DlpAssert struct(DlpCodeEnum ee) {
this.ee = ee;
return this;
}
public DlpAssert struct(String msg) {
this.ee = DlpCodeEnum.FAIL;
this.msg = msg;
return this;
}
public DlpAssert struct(DlpCodeEnum ee, String msg) {
this.ee = ee;
this.msg = msg;
return this;
}
public DlpAssert struct(DlpCodeEnum ee, String msg, Object data) {
this.ee = ee;
this.msg = msg;
this.data = data;
return this;
}
public DlpAssert withMsg(String msg) {
this.msg = msg;
return this;
}
public DlpAssert withData(Object obj) {
this.data = obj;
return this;
}
private DlpException factory() {
DlpException dlpException;
if(!Objects.isNull(msg)) {
dlpException = new DlpException(ee, msg);
} else {
dlpException = new DlpException(ee);
}
if (!Objects.isNull(data)) {
dlpException.setData(data);
}
return dlpException;
}
public void isThrow(boolean b) {
if(b) {
throw factory();
}
}
public void equals(String a, String b) {
if(!a.equals(b)) {
throw factory();
}
}
public void notNull(Object object) {
if (Objects.isNull(object)) {
throw factory();
}
}
public void notEmpty(Object object) {
if (null ==object) {
throw factory();
}
if (object instanceof Collection) {
int size = ((Collection) object).size();
if (size == 0) {
throw factory();
}
}
if (object.getClass().isArray()) {
int length = ((Object[]) object).length;
if (length == 0) {
throw factory();
}
}
}
}
枚举类
package com.toptoward.common.exception;
import java.util.ArrayList;
import java.util.List;
public enum DlpCodeEnum implements ExEnum {
CIRCUIT_BREAKER(1, "服务忙!"),
REPEAT_DEBOUNCE(10004, "请勿重复提交或者操作过于频繁!"),
SUCCESS(200,"执行成功"),
FAIL(201,"执行失败"),
FAIL_500(500,"执行失败"),
FAIL_10086(10086, "前端waring提示code"),
RES_401(401, "未登录或信息错误"),
RES_403(403, "授权已过期,无权限进行该操作!请重新导入授权。"),
RES_LOCK(10001, "账号已被锁定,请24小时后重试或联系用户管理员"),
PARAMETER_IS_EMTTY(10002, "参数为空"),
INVALID_PARAMETER(10003, "参数无效"),
;
public Integer code;
public String message;
DlpCodeEnum(Integer code, String message){
this.code = code;
this.message = message;
}
public Integer getCode(){
return code;
}
public String getMessage(){
return message;
}
}
使用
@demo DlpAssert.INSTANT.struct( DlpCodeEnum.FAIL).notNull(null);
@demo DlpAssert.INSTANT.struct(DlpCodeEnum.FAIL, "自定义异常信息").notNull(null);
@demo DlpAssert.INSTANT.withMsg("自定义异常信息").struct(DlpCodeEnum.FAIL).notNull(null);
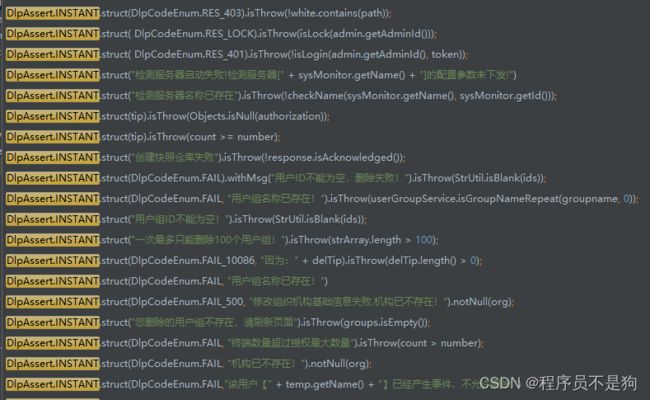
在web中全局捕捉
- GlobalExceptionHandler只能作用于进入controller的场景,否则不能捕捉,比如过滤器
- 如果AOP进行了异常捕捉,也不能进行捕捉
import java.util.Objects;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.security.access.AccessDeniedException;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import org.springframework.web.multipart.MaxUploadSizeExceededException;
import com.toptoward.common.exception.DlpCodeEnum;
import com.toptoward.common.exception.DlpException;
import com.toptoward.dlp.common.response.ResponseResult;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@RestControllerAdvice
public class GlobalExceptionHandler {
@Value("${spring.servlet.multipart.max-request-size}")
private String maxRequestSize;
@ExceptionHandler(DlpException.class)
public ResponseResult handleBizException(DlpException ex) {
log.error("from global 异常信息为: [{}]", ex.getMessage());
Integer code = DlpCodeEnum.DEFAULT_CODE.contains(ex.getErrCode()) ? ex.getErrCode()
: DlpCodeEnum.FAIL.getCode();
ResponseResult responseResult = new ResponseResult(code, ex.getMessage());
responseResult.setData(Objects.isNull(ex.getData())? "": ex.getData());
return responseResult;
}
@ExceptionHandler(AccessDeniedException.class)
public ResponseResult catchAccessDenied(AccessDeniedException ex) {
log.error("from global 异常信息为: [{}]", ex.getMessage());
return ResponseResult.failure("您的权限不足!");
}
@ExceptionHandler(Exception.class)
public Object exceptionHandler(Exception ex) {
log.error("异常信息为:", ex);
return ResponseResult.failure(DlpCodeEnum.CIRCUIT_BREAKER.getMessage());
}
@ExceptionHandler(value = MaxUploadSizeExceededException.class)
public ResponseResult handleBusinessException(MaxUploadSizeExceededException ex) {
log.error("上传文件异常信息:{}", ex.getMessage());
return ResponseResult.failure("上传文件大小超过最大限制:" + maxRequestSize);
}
}