坦克大战游戏
- 主函数
- 战场面板
- 开始界面
- 坦克父类
- 敌方坦克
- 我方坦克
- 子弹
- 爆炸效果
- 数据存盘及恢复
- 图片
主函数
package cn.wenxiao.release9;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
public class GameMain extends JFrame implements ActionListener {
WorldPanel mp = null;
StartPanel sPanel = null;
JMenuBar jBar = null;
JMenu menu1 = null;
JMenuItem startMenuItem = null;
JMenuItem exitGaMenuItem = null;
JMenuItem continueGaMenuItem = null;
JMenuItem exitWithoutSaveItem = null;
public static void main(String[] args) {
GameMain gameMain = new GameMain();
}
public GameMain() {
sPanel = new StartPanel();
jBar = new JMenuBar();
menu1 = new JMenu("游戏(G)");
menu1.setMnemonic('G');
startMenuItem = new JMenuItem("开始新游戏(N)");
startMenuItem.setMnemonic('N');
continueGaMenuItem = new JMenuItem("继续上一局游戏(C)");
continueGaMenuItem.setMnemonic('C');
exitGaMenuItem = new JMenuItem("保存并退出游戏(E)");
exitGaMenuItem.setMnemonic('E');
exitWithoutSaveItem = new JMenuItem("退出不保存游戏(Q)");
exitWithoutSaveItem.setActionCommand("quit");
exitWithoutSaveItem.addActionListener(this);
startMenuItem.addActionListener(this);
continueGaMenuItem.addActionListener(this);
exitGaMenuItem.addActionListener(this);
startMenuItem.setActionCommand("new_game");
continueGaMenuItem.setActionCommand("continue");
exitGaMenuItem.setActionCommand("exit");
menu1.add(startMenuItem);
menu1.add(continueGaMenuItem);
menu1.add(exitGaMenuItem);
menu1.add(exitWithoutSaveItem);
jBar.add(menu1);
this.add(sPanel);
Thread starThread = new Thread(sPanel);
starThread.start();
this.setJMenuBar(jBar);
this.setSize(800, 600);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equalsIgnoreCase("new_game")) {
this.remove(sPanel);
if (mp != null) {
this.remove(mp);
}
mp = new WorldPanel();
Recorder.setEnemyTankTotal(20);
Recorder.setKilledNum(0);
Recorder.setMyLife(3);
Thread mpThread = new Thread(mp);
mpThread.start();
this.add(mp);
this.addKeyListener(mp);
this.setVisible(true);
} else if (e.getActionCommand().equals("exit")) {
Recorder.saveData();
System.exit(0);
} else if (e.getActionCommand().equals("continue")) {
Recorder.readData();
this.remove(sPanel);
if (mp != null) {
this.remove(mp);
}
mp = new WorldPanel();
Thread mpThread = new Thread(mp);
mpThread.start();
this.add(mp);
this.addKeyListener(mp);
this.setVisible(true);
}else if(e.getActionCommand().equals("quit")) {
System.exit(0);
}
}
}
战场面板
package cn.wenxiao.release9;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.Toolkit;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Vector;
import javax.swing.JPanel;
public class WorldPanel extends JPanel implements KeyListener, Runnable {
MyTank myTank = null;
int enemyTankbirth = 0;
Vector<EnemyTank> enemyTanks = new Vector<EnemyTank>();
Vector<Bomb> bombs = new Vector<Bomb>();
Image image1 = null;
Image image2 = null;
Image image3 = null;
int enemyCounts = 3;
public WorldPanel() {
myTank = new MyTank(260, 380, 0);
image1 = Toolkit.getDefaultToolkit().getImage(WorldPanel.class.getResource("/3.png"));
image2 = Toolkit.getDefaultToolkit().getImage(WorldPanel.class.getResource("/2.png"));
image3 = Toolkit.getDefaultToolkit().getImage(WorldPanel.class.getResource("/1.png"));
}
public void paint(Graphics graphics) {
super.paint(graphics);
graphics.fillRect(0, 0, 600, 400);
for (int i = 0; i < bombs.size(); i++) {
Bomb curentBomb = bombs.get(i);
if (curentBomb.life > 5) {
graphics.drawImage(image1, curentBomb.x, curentBomb.y, 30, 30, this);
} else if (bombs.get(i).life > 3) {
graphics.drawImage(image2, curentBomb.x, curentBomb.y, 30, 30, this);
} else {
graphics.drawImage(image3, curentBomb.x, curentBomb.y, 30, 30, this);
}
curentBomb.lifeDown();
if (curentBomb.life < 0) {
bombs.remove(curentBomb);
}
}
if (myTank.isAlive) {
this.drawTank(myTank.getX(), myTank.getY(), graphics, this.myTank.getDirect(), 0);
}
for (int i = 0; i < enemyTanks.size(); i++) {
EnemyTank currentTank = enemyTanks.get(i);
if (currentTank.isAlive) {
this.drawTank(currentTank.getX(), currentTank.getY(), graphics, currentTank.direct, 1);
}
if (currentTank.isAlive == false) {
enemyTanks.remove(i);
}
if (currentTank.bullets.size() > 0) {
for (int j = 0; j < currentTank.bullets.size(); j++) {
Bullet curBullet = currentTank.bullets.get(j);
if (curBullet != null && curBullet.isAlive == true) {
graphics.draw3DRect(curBullet.x, curBullet.y, 1, 1, false);
}
if (curBullet.isAlive == false) {
currentTank.bullets.remove(curBullet);
}
}
}
}
for (int i = 0; i < myTank.bullets.size(); i++) {
Bullet currBullet = myTank.bullets.get(i);
if (currBullet != null && currBullet.isAlive == true) {
graphics.draw3DRect(currBullet.x, currBullet.y, 1, 1, false);
}
if (currBullet.isAlive == false) {
myTank.bullets.remove(currBullet);
}
}
this.paintInfo(graphics);
}
public void paintInfo(Graphics graphics) {
this.drawTank(20, 430, graphics, 0, 1);
this.drawTank(20, 480, graphics, 0, 0);
graphics.setColor(Color.BLACK);
Font font = new Font("微软雅黑", Font.BOLD, 20);
graphics.setFont(font);
graphics.drawString("剩余:" + Recorder.getEnemyTankTotal(), 40, 440);
graphics.drawString("剩余:" + Recorder.getMyLife(), 40, 480);
graphics.drawString("已击杀: " + Recorder.getKilledNum(), 610, 30);
}
public void beHited(MyTank myTank, Vector<EnemyTank> enemyTanks) {
for (int i = 0; i < this.enemyTanks.size(); i++) {
EnemyTank enemyTank = enemyTanks.get(i);
for (int j = 0; j < enemyTank.bullets.size(); j++) {
Bullet currentBullet = enemyTank.bullets.get(j);
switch (myTank.direct) {
case 0:
case 2:
if (currentBullet.x > myTank.getX() - 10 && currentBullet.x < myTank.getX() + 10
&& currentBullet.y > myTank.getY() - 15 && currentBullet.y < myTank.getY() + 15) {
Bomb bomb = new Bomb(myTank.getX() - 15, myTank.getY() - 15);
bombs.add(bomb);
currentBullet.isAlive = false;
myTank.isAlive = false;
Recorder.myLifeDown();
}
break;
case 1:
case 3:
if (currentBullet.x > myTank.getX() - 15 && currentBullet.x < myTank.getX() + 15
&& currentBullet.y > myTank.getY() - 10 && currentBullet.y < myTank.getY() + 10) {
Bomb bomb = new Bomb(myTank.getX() - 5, myTank.getY() - 5);
bombs.add(bomb);
currentBullet.isAlive = false;
myTank.isAlive = false;
Recorder.myLifeDown();
}
break;
}
}
}
}
public void hited(Bullet bullet, EnemyTank enemyTank) {
switch (enemyTank.direct) {
case 0:
case 2:
if (bullet.x > enemyTank.getX() - 10 && bullet.x < enemyTank.getX() + 10 && bullet.y > enemyTank.getY() - 15
&& bullet.y < enemyTank.getY() + 15) {
Bomb bomb = new Bomb(enemyTank.getX() - 15, enemyTank.getY() - 15);
bombs.add(bomb);
bullet.isAlive = false;
enemyTank.isAlive = false;
Recorder.enemyTankTotalDown();
Recorder.killedNumUp();
}
break;
case 1:
case 3:
if (bullet.x > enemyTank.getX() - 15 && bullet.x < enemyTank.getX() + 15 && bullet.y > enemyTank.getY() - 10
&& bullet.y < enemyTank.getY() + 10) {
Bomb bomb = new Bomb(enemyTank.getX() - 5, enemyTank.getY() - 5);
bombs.add(bomb);
bullet.isAlive = false;
enemyTank.isAlive = false;
Recorder.enemyTankTotalDown();
Recorder.killedNumUp();
}
break;
}
}
public void drawTank(int x, int y, Graphics graphics, int direct, int type) {
switch (type) {
case 0:
graphics.setColor(Color.CYAN);
break;
case 1:
graphics.setColor(Color.YELLOW);
break;
}
switch (direct) {
case 0:
graphics.fill3DRect(x - 10, y - 15, 5, 30, false);
graphics.fill3DRect(x + 5, y - 15, 5, 30, false);
graphics.fill3DRect(x - 5, y - 10, 10, 20, false);
graphics.fillOval(x - 5, y - 5, 10, 10);
graphics.drawLine(x, y - 15, x, y);
break;
case 1:
graphics.fill3DRect(x - 15, y - 10, 30, 5, false);
graphics.fill3DRect(x - 15, y + 5, 30, 5, false);
graphics.fill3DRect(x - 10, y - 5, 20, 10, false);
graphics.fillOval(x - 5, y - 5, 10, 10);
graphics.drawLine(x, y, x + 15, y);
break;
case 2:
graphics.fill3DRect(x - 10, y - 15, 5, 30, false);
graphics.fill3DRect(x + 5, y - 15, 5, 30, false);
graphics.fill3DRect(x - 5, y - 10, 10, 20, false);
graphics.fillOval(x - 5, y - 5, 10, 10);
graphics.drawLine(x, y, x, y + 15);
break;
case 3:
graphics.fill3DRect(x - 15, y - 10, 30, 5, false);
graphics.fill3DRect(x - 15, y + 5, 30, 5, false);
graphics.fill3DRect(x - 10, y - 5, 20, 10, false);
graphics.fillOval(x - 5, y - 5, 10, 10);
graphics.drawLine(x - 15, y, x, y);
break;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_W) {
this.myTank.setDirect(0);
this.myTank.moveUp();
} else if (e.getKeyCode() == KeyEvent.VK_D) {
this.myTank.setDirect(1);
this.myTank.moveRight();
} else if (e.getKeyCode() == KeyEvent.VK_S) {
this.myTank.setDirect(2);
this.myTank.moveDown();
} else if (e.getKeyCode() == KeyEvent.VK_A) {
this.myTank.setDirect(3);
this.myTank.moveLeft();
}
if (e.getKeyCode() == KeyEvent.VK_J) {
if (myTank.bullets.size() < 5) {
this.myTank.Shot();
}
}
开始界面
package cn.wenxiao.release9;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import javax.swing.JPanel;
public class StartPanel extends JPanel implements Runnable{
int times=0;
public void paint(Graphics graphics) {
super.paint(graphics);
graphics.fillRect(0, 0, 600, 400);
graphics.setColor(Color.YELLOW);
Font myFont = new Font("微软雅黑",Font.BOLD,50);
graphics.setFont(myFont);
if(times%2==0) {
graphics.drawString("第一关", 230, 210);
}
}
@Override
public void run() {
while(true) {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
this.repaint();
times++;
if(times>10000) {
times=0;
}
}
}
}
坦克父类
package cn.wenxiao.release9;
public class Tanks {
int x = 0;
int y = 0;
int direct = 0;
int color;
public int getColor() {
return color;
}
public void setColor(int color) {
this.color = color;
}
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
int speed = 2;
public Tanks(int x, int y, int direct) {
this.x = x;
this.y = y;
this.direct = direct;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getDirect() {
return direct;
}
public void setDirect(int direct) {
this.direct = direct;
}
}
敌方坦克
package cn.wenxiao.release9;
import java.util.Vector;
public class EnemyTank extends Tanks implements Runnable {
boolean isAlive = true;
int direct = 2;
Bullet bullet = null;
Vector<Bullet> bullets = new Vector<Bullet>();
Vector<EnemyTank> enemyTanks = new Vector<EnemyTank>();
public Vector<EnemyTank> getEnemyTanks() {
return enemyTanks;
}
public void setEnemyTanks(Vector<EnemyTank> enemyTanks) {
this.enemyTanks = enemyTanks;
}
public EnemyTank(int x, int y, int direct) {
super(x, y, direct);
}
@Override
public String toString() {
return "EnemyTank [isAlive=" + isAlive + ", direct=" + direct + "]";
}
public boolean isOverlapped() {
boolean status = false;
switch(this.direct) {
case 0:
for(int i=0;i<enemyTanks.size();i++) {
EnemyTank thEnemyTank = enemyTanks.get(i);
if(thEnemyTank!=this) {
if(thEnemyTank.direct==0 || thEnemyTank.direct==2) {
if((this.x-10)>=(thEnemyTank.x-10) && (this.x-10)<=(thEnemyTank.x+10) && (this.y-15) >= (thEnemyTank.y-15) && (this.y-15)<=(thEnemyTank.y+15)) {
return true;
}
if((this.x+10)>=(thEnemyTank.x-10) && (this.x+10)<=(thEnemyTank.x+10) && (this.y-15) >= (thEnemyTank.y-15) && (this.y-15)<=(thEnemyTank.y+15)) {
return true;
}
}
if(thEnemyTank.direct==1 || thEnemyTank.direct==3) {
if((this.x-10)>=(thEnemyTank.x-15) && (this.x-10)<=(thEnemyTank.x+15) && (this.y-15) >= (thEnemyTank.y-15) && (this.y-15)<=(thEnemyTank.y+15)) {
return true;
}
if((this.x+10)>=(thEnemyTank.x-15) && (this.x+10)<=(thEnemyTank.x+15) && (this.y-15) >= (thEnemyTank.y-10) && (this.y-15)<=(thEnemyTank.y+15)) {
return true;
}
}
}
}
break;
case 1:
for(int i=0;i<enemyTanks.size();i++) {
EnemyTank thEnemyTank = enemyTanks.get(i);
if(thEnemyTank!=this) {
if(thEnemyTank.direct==0 || thEnemyTank.direct==2) {
if((this.x+15)>=(thEnemyTank.x-10) && (this.x+15)<=(thEnemyTank.x-10) && (this.y-10) >= (thEnemyTank.y-15) && (this.y-10)<=(thEnemyTank.y+15)) {
return true;
}
if((this.x+15)>=(thEnemyTank.x-10) && (this.x+15)<=(thEnemyTank.x+10) && (this.y-10) >= (thEnemyTank.y+15) && (this.y+10)<=(thEnemyTank.y+15)) {
return true;
}
}
if(thEnemyTank.direct==1 || thEnemyTank.direct==3) {
if((this.x+15)>=(thEnemyTank.x-15) && (this.x+15)<=(thEnemyTank.x+15) && (this.y-10) >= (thEnemyTank.y-10) && (this.y-10)<=(thEnemyTank.y+10)) {
return true;
}
if((this.x+15)>=(thEnemyTank.x-15) && (this.x+15)<=(thEnemyTank.x+15) && (this.y+10) >= (thEnemyTank.y-10) && (this.y+10)<=(thEnemyTank.y+10)) {
return true;
}
}
}
}
break;
case 2:
for(int i=0;i<enemyTanks.size();i++) {
EnemyTank thEnemyTank = enemyTanks.get(i);
if(thEnemyTank!=this) {
if(thEnemyTank.direct==0 || thEnemyTank.direct==2) {
if((this.x-10)>=(thEnemyTank.x-10) && (this.x-10)<=(thEnemyTank.x+10) && (this.y+15) >= (thEnemyTank.y-15) && (this.y+15)<=(thEnemyTank.y+15)) {
return true;
}
if((this.x+10)>=(thEnemyTank.x-10) && (this.x+10)<=(thEnemyTank.x+10) && (this.y+15) >= (thEnemyTank.y-15) && (this.y+15)<=(thEnemyTank.y+15)) {
return true;
}
}
if(thEnemyTank.direct==1 || thEnemyTank.direct==3) {
if((this.x-10)>=(thEnemyTank.x-15) && (this.x-10)<=(thEnemyTank.x+15) && (this.y+15) >= (thEnemyTank.y-10) && (this.y+15)<=(thEnemyTank.y+10)) {
return true;
}
if((this.x+10)>=(thEnemyTank.x-10) && (this.x+10)<=(thEnemyTank.x+15) && (this.y+15) >= (thEnemyTank.y-10) && (this.y+15)<=(thEnemyTank.y+10)) {
return true;
}
}
}
}
break;
case 3:
for(int i=0;i<enemyTanks.size();i++) {
EnemyTank thEnemyTank = enemyTanks.get(i);
if(thEnemyTank!=this) {
if(thEnemyTank.direct==0 || thEnemyTank.direct==2) {
if((this.x-15)>=(thEnemyTank.x-10) && (this.x-15)<=(thEnemyTank.x+10) && (this.y-10) >= (thEnemyTank.y-15) && (this.y-10)<=(thEnemyTank.y+15)) {
return true;
}
if((this.x-15)>=(thEnemyTank.x-10) && (this.x-15)<=(thEnemyTank.x+10) && (this.y+10) >= (thEnemyTank.y-15) && (this.y+10)<=(thEnemyTank.y+15)) {
return true;
}
}
if(thEnemyTank.direct==1 || thEnemyTank.direct==3) {
if((this.x-15)>=(thEnemyTank.x-15) && (this.x-15)<=(thEnemyTank.x+15) && (this.y-10) >= (thEnemyTank.y-10) && (this.y-10)<=(thEnemyTank.y+10)) {
return true;
}
if((this.x-15)>=(thEnemyTank.x-15) && (this.x-15)<=(thEnemyTank.x+15) && (this.y+10) >= (thEnemyTank.y-10) && (this.y+10)<=(thEnemyTank.y+10)) {
return true;
}
}
}
}
break;
}
return status;
}
public void moveUp() {
y -= speed;
}
public void moveRight() {
x += speed;
}
public void moveDown() {
y += speed;
}
public void moveLeft() {
x -= speed;
}
public void Shot() {
switch (direct) {
case 0:
bullet = new Bullet(x - 1, y - 15, 0);
bullets.add(bullet);
break;
case 1:
bullet = new Bullet(x + 15, y, 1);
bullets.add(bullet);
break;
case 2:
bullet = new Bullet(x - 1, y + 15, 2);
bullets.add(bullet);
break;
case 3:
bullet = new Bullet(x - 15, y, 3);
bullets.add(bullet);
break;
}
Thread shoThread = new Thread(bullet);
shoThread.start();
}
@Override
public void run() {
while (true) {
if (x > 15 && x < 570 && y > 15 && y < 450 && !this.isOverlapped()) {
int RandomShot = (int) (Math.random() * 100);
if (RandomShot < 50) {
this.Shot();
}
}
switch (this.direct) {
case 0:
for (int i = 0; i < 30; i++) {
if (y > 15 && !this.isOverlapped()) {
y -= speed;
}
try {
Thread.sleep(50);
} catch (Exception e) {
e.printStackTrace();
}
}
break;
case 1:
for (int i = 0; i < 30; i++) {
if (x < 450 && !this.isOverlapped()) {
x += speed;
}
try {
Thread.sleep(50);
} catch (Exception e) {
e.printStackTrace();
}
}
break;
case 2:
for (int i = 0; i < 30; i++) {
if (y < 380 && !this.isOverlapped()) {
y += speed;
}
try {
Thread.sleep(50);
} catch (Exception e) {
e.printStackTrace();
}
}
break;
case 3:
for (int i = 0; i < 30; i++) {
if (x > 15 && !this.isOverlapped()) {
x -= speed;
}
try {
Thread.sleep(50);
} catch (Exception e) {
e.printStackTrace();
}
}
break;
}
this.direct = (int) (Math.random() * 4);
if (isAlive == false) {
break;
}
}
}
}
我方坦克
package cn.wenxiao.release9;
import java.util.Vector;
public class MyTank extends Tanks {
Bullet bullet = null;
Vector<Bullet> bullets = new Vector<Bullet>();
boolean isAlive = true;
public MyTank(int x, int y, int direct) {
super(x, y, direct);
}
public void Shot() {
switch (direct) {
case 0:
bullet = new Bullet(x - 1, y - 15, 0);
bullets.add(bullet);
break;
case 1:
bullet = new Bullet(x + 15, y, 1);
bullets.add(bullet);
break;
case 2:
bullet = new Bullet(x - 1, y + 15, 2);
bullets.add(bullet);
break;
case 3:
bullet = new Bullet(x - 15, y, 3);
bullets.add(bullet);
break;
}
Thread shoThread = new Thread(bullet);
shoThread.start();
}
public void moveUp() {
if (y > 15) {
y -= speed;
}
}
public void moveRight() {
if (x < 770) {
x += speed;
}
}
public void moveDown() {
if (y < 550) {
y += speed;
}
}
public void moveLeft() {
if (x > 15) {
x -= speed;
}
}
}
子弹
package cn.wenxiao.release9;
public class Bullet implements Runnable{
int x;
int y;
int directory;
int speed = 10;
Boolean isAlive = true;
public Bullet(int x, int y, int directory) {
this.x = x;
this.y = y;
this.directory = directory;
}
@Override
public void run() {
while(true) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
switch(directory) {
case 0:
y -= speed;
break;
case 1:
x += speed;
break;
case 2:
y += speed;
break;
case 3:
x -= speed;
break;
}
if(x<0 || x >600 || y < 0 || y>400) {
this.isAlive = false;
break;
}
}
}
}
爆炸效果
package cn.wenxiao.release9;
public class Bomb {
int x;
int y;
int life=8;
boolean isAlive=true;
public Bomb(int x, int y) {
this.x = x;
this.y = y;
}
public void lifeDown() {
if(life>=0) {
life--;
}else {
isAlive=false;
}
}
}
数据存盘及恢复
package cn.wenxiao.release9;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Vector;
public class Recorder {
private static int enemyTankTotal = 20;
private static int myLife = 3;
private static int killedNum = 0;
private static FileWriter fWriter = null;
private static BufferedWriter bWriter = null;
private static FileReader fReader = null;
private static BufferedReader bReader = null;
private static Vector<EnemyTank> enemyTanks = null;
private static boolean isContinue = false;
public static Vector<EnemyTank> getEnemyTanks() {
return enemyTanks;
}
public static void setEnemyTanks(Vector<EnemyTank> enemyTanks) {
Recorder.enemyTanks = enemyTanks;
}
public static int getEnemyTankTotal() {
return enemyTankTotal;
}
public static void setEnemyTankTotal(int enemyTankTotal) {
Recorder.enemyTankTotal = enemyTankTotal;
}
public static int getMyLife() {
return myLife;
}
public static void setMyLife(int myLife) {
Recorder.myLife = myLife;
}
public static int getKilledNum() {
return killedNum;
}
public static void setKilledNum(int killedNum) {
Recorder.killedNum = killedNum;
}
public static boolean isContinue() {
return isContinue;
}
public static void setContinue(boolean isContinue) {
Recorder.isContinue = isContinue;
}
public static void saveData() {
try {
fWriter = new FileWriter(".\\GameData.txt");
bWriter = new BufferedWriter(fWriter);
bWriter.write("当前总击杀:" + killedNum + "\r\n");
bWriter.write("敌机剩余:" + enemyTankTotal + "\r\n");
bWriter.write("我的生命剩余:" + myLife + "\r\n");
for (int i = 0; i < enemyTanks.size(); i++) {
EnemyTank currEnemyTank = enemyTanks.get(i);
String positionData = "第" + (i + 1) + "辆敌机坐标:" + "X:" + currEnemyTank.x + ":Y:" + currEnemyTank.y
+ ":Directory:" + currEnemyTank.direct + "\r\n";
bWriter.write(positionData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
bWriter.close();
fWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void readData() {
enemyTanks = new Vector<EnemyTank>();
isContinue = true;
try {
fReader = new FileReader(".\\GameData.txt");
bReader = new BufferedReader(fReader);
int[] data = new int[3];
int index = 0;
String dataString = null;
while ((dataString = bReader.readLine()) != null) {
String[] string = dataString.split(":");
if (index < 3) {
data[index++] = Integer.parseInt(string[1]);
} else if (index >= 3) {
int x = Integer.parseInt(string[2]);
int y = Integer.parseInt(string[4]);
int direct = Integer.parseInt(string[6]);
EnemyTank enemyTank = new EnemyTank(x, y, direct);
enemyTanks.add(enemyTank);
}
}
killedNum = data[0];
enemyTankTotal = data[1];
myLife = data[2];
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
bReader.close();
fReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static void enemyTankTotalDown() {
enemyTankTotal--;
}
public static void myLifeDown() {
myLife--;
}
public static void killedNumUp() {
killedNum++;
}
}
图片
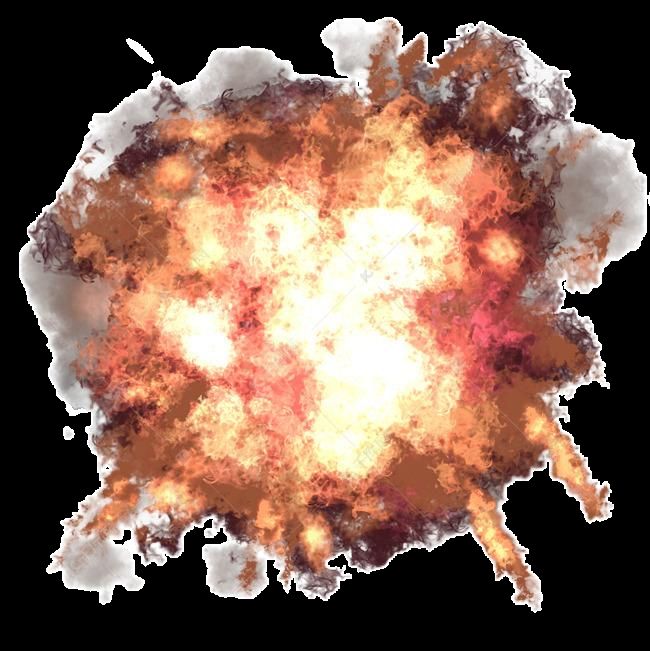
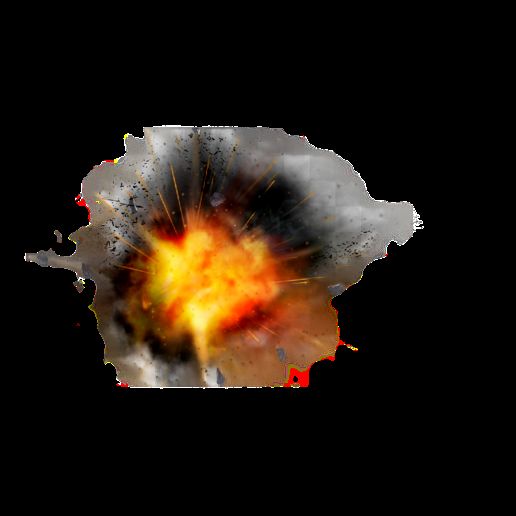
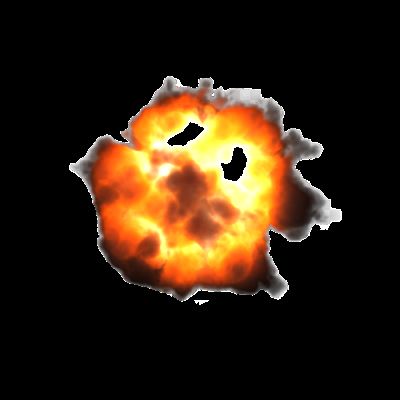